Bob has an array of n integers. Output the number of distinct values in the array . Input The first input line has an integer n: the number of values. The second line has n integers x1,x2,…,xn. Output Print one integers: the number of distinct values. Constraints 1≤n≤2⋅10*5 1≤xi≤10*9
时间: 2024-03-12 14:43:22 浏览: 45
To solve this problem, we can use a set to keep track of all the distinct values in the array. We can iterate through the array and add each element to the set. At the end, the size of the set will give us the number of distinct values.
Here's the Python code to solve the problem:
```
n = int(input())
arr = list(map(int, input().split()))
distinct_values = set()
for i in range(n):
distinct_values.add(arr[i])
print(len(distinct_values))
```
This code reads in the input, creates an empty set called `distinct_values`, and then iterates through the array `arr`, adding each element to the set. Finally, it prints the size of the set using the `len()` function.
相关问题
Bob has an array of � n integers. Output the number of distinct values in the array . Input The first input line has an integer � n: the number of values. The second line has � n integers � 1 , � 2 , … , � � x 1 ,x 2 ,…,x n . Output Print one integers: the number of distinct values. Constraints 1 ≤ � ≤ 2 ⋅ 1 0 5 1≤n≤2⋅10 5 1 ≤ � � ≤ 1 0 9 1≤x i ≤10 9
这道题可以使用哈希表(Hash Table)来解决。我们可以遍历整个数组,将每个元素作为键(key)插入哈希表中,如果哈希表中已经存在该键,则不进行任何操作,如果哈希表中不存在该键,则将该键插入哈希表中,并将计数器加一。
最后,哈希表中的键的数量就是数组中不同值的数量。
以下是Python的实现代码:
```
n = int(input())
a = list(map(int, input().split()))
unique_values = set()
for x in a:
unique_values.add(x)
print(len(unique_values))
```
You are given two arrays a and b each consisting of n integers. All elements of a are pairwise distinct. Find the number of ways to reorder a such that ai>bi for all 1≤i≤n , modulo 109+7 . Two ways of reordering are considered different if the resulting arrays are different. Input Each test contains multiple test cases. The first line contains the number of test cases t (1≤t≤104 ). The description of the test cases follows. The first line of each test case contains a single integer n (2≤n≤2⋅105 ) — the length of the array a and b . The second line of each test case contains n distinct integers a1 , a2 , … , an (1≤ai≤109 ) — the array a . It is guaranteed that all elements of a are pairwise distinct. The second line of each test case contains n integers b1 , b2 , … , bn (1≤bi≤109 ) — the array b . It is guaranteed that the sum of n over all test cases does not exceed 2⋅105 . Output For each test case, output the number of ways to reorder array a such that ai>bi for all 1≤i≤n , modulo 109+7 .
题意简述:给定两个长度为n的数组a和b,每个数组都由n个不同的正整数组成。找出重新排列数组a的方式,使得对于所有1≤i≤n,ai>bi。如果得到的数组不同,则认为两种重新排列方式是不同的。求满足条件的方案数,对109+7取模。
思路:贪心+逆序对
首先,对数组a进行升序排序,对数组b进行降序排序。
然后,从a数组的末尾开始遍历,对于每个a[i],找到b中第一个小于a[i]的数字b[j],将a[i]插入到b[j]的前面,这样能够保证ai>bi,同时不改变其他数字的相对顺序。
最后,统计逆序对的个数即可,因为只有在构造过程中出现的逆序对才能够影响到方案数。
时间复杂度:O(nlogn)
参考代码:
阅读全文
相关推荐
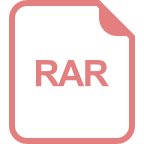
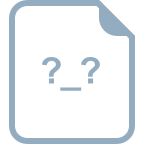
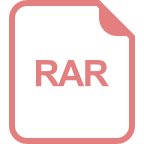












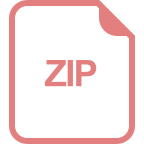
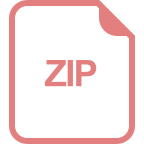