月薪6000,试用期间薪资4800,当月22号转正,按照30天计算总工时为21.75,分开计算的话,当月应该发多少工资?
时间: 2024-10-25 12:18:15 浏览: 24
如果员工的工资结构包括基本工资和可能的绩效奖金部分,那么试用期和转正后的薪资计算可能会有所不同。假设月薪6000元是全月的基本工资,试用期薪资4800元代表的是按比例发放的基础部分。
**试用期工资计算**(基于21.75天工作时间):
```python
# 计算每日工资
daily_wage = 4800 / 21.75
# 当月实际工作天数
actual_days = 22
# 试用期内工资总额
salary_during_trial = daily_wage * actual_days
```
**转正后的工资计算**(剩余的8.25天):
由于22号转正,我们可以假设剩余的8.25天属于正式员工工资计算范围,但是具体的奖金或其他福利部分没有提及,这里我们只考虑基础工资的计算:
```python
# 转正后的工作天数
post_trial_days = 30 - 22
# 转正后工资总额(仅基本工资)
salary_after_trial = 6000 * (post_trial_days / 30)
```
**合计**:
试用期工资 + 转正后工资 = 总工资
请注意,这只是一个基本的计算框架,实际情况下可能还有扣税、社保、公积金等其他因素需要考虑。如果你能提供更多关于薪酬结构的信息,可以得到更精确的结果。
相关问题
某公司雇员(employee)包括经理(manager),技术人员(technician)和销售员(salesman)。开发部经理(developermanger),既是经理也是技术人员。销售部经理(salesmanager),既是经理也是销售员。 以employ类为虚基类派生出manager,technician和salesman类;再进一步派生出developermanager和salesmanager类。 employee类的属性包括姓名、职工号、工资级别,月薪(实发基本工资加业绩工资)。操作包括月薪计算函数(pay()),该函数要求输入请假天数,扣去应扣工资后,得出实发基本工资。 technician类派生的属性有每小时附加酬金和当月工作时数,及研究完成进度系数。业绩工资为三者之积。也包括同名的pay()函数,工资总额为基本工资加业绩工资。 salesman类派生的属性有当月销售额和酬金提取百分比,业绩工资为两者之积。也包括同名的pay()函数,工资总额为基本工资加业绩工资。 manager类派生属性有固定奖金额和业绩系数,业绩工资为两者之积。工资总额也为基本工资加业绩工资。 而developermanager类,pay()函数是将作为经理和作为技术人员业绩工资之和的一半作为业绩工资。 salesamanager类,pay()函数则是经理的固定奖金额的一半,加上部门总销售额与提成比例之积,这是业绩工资。 编程实现工资管理。特别注意pay()的定义和调用方法:先用同名覆盖,再用运行时多态。
好的,这是一个比较复杂的继承关系,需要仔细设计类和函数。下面是一个基本的实现,可能还需要根据具体需求进行修改:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Employee {
protected:
string name;
int id;
int level;
double base_salary;
double performance_salary;
public:
Employee(string name, int id, int level):
name(name), id(id), level(level), base_salary(0), performance_salary(0) {}
virtual double pay(int days) {
base_salary = level * 100; // 假设基本工资为级别乘以100元
double deducted_salary = days * base_salary / 21.75; // 假设每月工作日为21.75天
base_salary -= deducted_salary;
return base_salary + performance_salary;
}
};
class Manager: virtual public Employee {
protected:
double fixed_bonus;
double performance_factor;
public:
Manager(string name, int id, int level, double fixed_bonus, double performance_factor):
Employee(name, id, level), fixed_bonus(fixed_bonus), performance_factor(performance_factor) {}
virtual double pay(int days) {
base_salary = level * 100; // 假设基本工资为级别乘以100元
performance_salary = base_salary * performance_factor + fixed_bonus;
double deducted_salary = days * base_salary / 21.75; // 假设每月工作日为21.75天
base_salary -= deducted_salary;
return base_salary + performance_salary;
}
};
class Technician: virtual public Employee {
protected:
double hourly_wage;
int working_hours;
double progress_factor;
public:
Technician(string name, int id, int level, double hourly_wage, int working_hours, double progress_factor):
Employee(name, id, level), hourly_wage(hourly_wage), working_hours(working_hours), progress_factor(progress_factor) {}
virtual double pay(int days) {
base_salary = level * 100; // 假设基本工资为级别乘以100元
performance_salary = base_salary * hourly_wage * working_hours * progress_factor;
double deducted_salary = days * base_salary / 21.75; // 假设每月工作日为21.75天
base_salary -= deducted_salary;
return base_salary + performance_salary;
}
};
class Salesman: virtual public Employee {
protected:
double sales_amount;
double commission_rate;
public:
Salesman(string name, int id, int level, double sales_amount, double commission_rate):
Employee(name, id, level), sales_amount(sales_amount), commission_rate(commission_rate) {}
virtual double pay(int days) {
base_salary = level * 100; // 假设基本工资为级别乘以100元
performance_salary = base_salary * sales_amount * commission_rate;
double deducted_salary = days * base_salary / 21.75; // 假设每月工作日为21.75天
base_salary -= deducted_salary;
return base_salary + performance_salary;
}
};
class DeveloperManager: public Manager, public Technician {
public:
DeveloperManager(string name, int id, int level, double fixed_bonus, double performance_factor, double hourly_wage, int working_hours, double progress_factor):
Employee(name, id, level), Manager(name, id, level, fixed_bonus, performance_factor), Technician(name, id, level, hourly_wage, working_hours, progress_factor) {}
virtual double pay(int days) {
base_salary = level * 100; // 假设基本工资为级别乘以100元
performance_salary = (base_salary * performance_factor + fixed_bonus) / 2 + base_salary * hourly_wage * working_hours * progress_factor / 2;
double deducted_salary = days * base_salary / 21.75; // 假设每月工作日为21.75天
base_salary -= deducted_salary;
return base_salary + performance_salary;
}
};
class SalesManager: public Manager, public Salesman {
public:
SalesManager(string name, int id, int level, double fixed_bonus, double performance_factor, double sales_amount, double commission_rate):
Employee(name, id, level), Manager(name, id, level, fixed_bonus, performance_factor), Salesman(name, id, level, sales_amount, commission_rate) {}
virtual double pay(int days) {
base_salary = level * 100; // 假设基本工资为级别乘以100元
performance_salary = fixed_bonus / 2 + base_salary * sales_amount * commission_rate;
double deducted_salary = days * base_salary / 21.75; // 假设每月工作日为21.75天
base_salary -= deducted_salary;
return base_salary + performance_salary;
}
};
int main() {
Employee* employee1 = new Technician("John", 1001, 3, 5, 160, 0.8);
Employee* employee2 = new Salesman("Mary", 1002, 4, 10000, 0.02);
Employee* employee3 = new Manager("Tom", 1003, 5, 5000, 0.1);
Employee* employee4 = new DeveloperManager("Jack", 1004, 6, 10000, 0.2, 6, 200, 1.2);
Employee* employee5 = new SalesManager("Lucy", 1005, 7, 8000, 0.15, 20000, 0.01);
cout << employee1->pay(5) << endl;
cout << employee2->pay(10) << endl;
cout << employee3->pay(15) << endl;
cout << employee4->pay(20) << endl;
cout << employee5->pay(25) << endl;
delete employee1;
delete employee2;
delete employee3;
delete employee4;
delete employee5;
return 0;
}
```
在上面的代码中,我们定义了五个类:`Employee`、`Manager`、`Technician`、`Salesman`、`DeveloperManager` 和`SalesManager`。其中,`Employee`是虚基类,其他类都从它派生而来。每个类都有自己的属性和函数,其中`pay()`函数是重点,用于计算工资。在`DeveloperManager`和`SalesManager`中,我们使用了虚基类,同时需要注意避免多次继承问题。最后,在`main()`函数中,我们创建了五个对象,并分别调用它们的`pay()`函数来计算工资。
用c++计算员工工资
好的,下面是一个简单的 C++ 代码示例,计算员工工资:
```c++
#include <iostream>
using namespace std;
int main() {
// 输入员工基本工资和加班时长
double base_salary, overtime_hours;
cout << "请输入员工基本工资:";
cin >> base_salary;
cout << "请输入员工加班时长:";
cin >> overtime_hours;
// 计算加班工资和总工资
double overtime_salary = overtime_hours * 1.5 * (base_salary / 21.75);
double total_salary = base_salary + overtime_salary;
// 输出结果
cout << "员工基本工资为:" << base_salary << endl;
cout << "员工加班工资为:" << overtime_salary << endl;
cout << "员工总工资为:" << total_salary << endl;
return 0;
}
```
这个示例中,我们假设员工的每月工作时间为 21.75 天,每小时加班工资为基本工资的 1.5 倍。程序会要求输入员工的基本工资和加班时长,然后计算出加班工资和总工资,并输出结果。
阅读全文
相关推荐
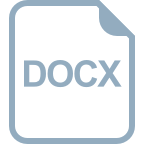
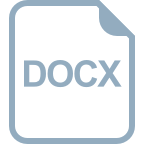
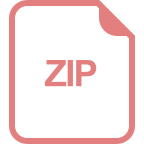
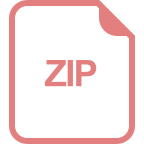
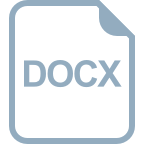
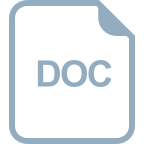
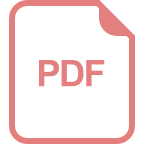
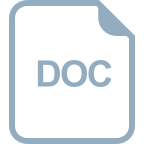
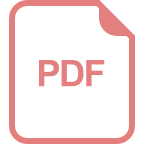
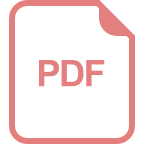
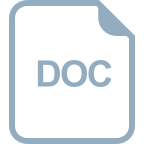
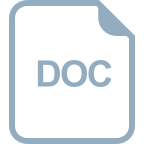



