手动实现Delaunay三角剖分算法
时间: 2023-10-10 15:11:54 浏览: 221
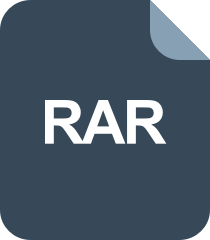
Delaunay三角剖分算法 C++
Delaunay三角剖分算法是一种常用的二维平面三角形剖分方法,常用于计算机图形学、地理信息系统、计算几何等领域。下面是一种手动实现的Delaunay三角剖分算法,供参考。
1. 输入点集P,初始化三角形集T为一个超级三角形
2. 将P中每个点插入到T中
3. 对于P中每个点p,查找在T中包含p的三角形t
4. 将t的三条边删除,得到三个新的三角形,如果这些三角形不满足Delaunay条件,则进行翻转操作
5. 将新的三角形加入T中
6. 删除T中所有与超级三角形相邻的三角形
以下是Python代码实现:
```python
from typing import List, Tuple
class Triangle:
def __init__(self, points: List[Tuple[float, float]]):
self.points = points
self.circumcircle = self.get_circumcircle()
def get_circumcircle(self):
x1, y1 = self.points[0]
x2, y2 = self.points[1]
x3, y3 = self.points[2]
D = (x1 - x2) * (y2 - y3) - (x2 - x3) * (y1 - y2)
if abs(D) < 1e-8:
return None
x = ((x1 ** 2 + y1 ** 2) * (y2 - y3) + (x2 ** 2 + y2 ** 2) * (y3 - y1) + (x3 ** 2 + y3 ** 2) * (y1 - y2)) / (2 * D)
y = -((x1 ** 2 + y1 ** 2) * (x2 - x3) + (x2 ** 2 + y2 ** 2) * (x3 - x1) + (x3 ** 2 + y3 ** 2) * (x1 - x2)) / (2 * D)
r = ((x - x1) ** 2 + (y - y1) ** 2) ** 0.5
return (x, y, r)
def contains(self, point: Tuple[float, float]):
x, y = point
x1, y1 = self.points[0]
x2, y2 = self.points[1]
x3, y3 = self.points[2]
if (y2 - y1) * (x - x1) - (x2 - x1) * (y - y1) < 0:
return False
if (y3 - y2) * (x - x2) - (x3 - x2) * (y - y2) < 0:
return False
if (y1 - y3) * (x - x3) - (x1 - x3) * (y - y3) < 0:
return False
return True
def flip(self):
p1, p2, p3 = self.points
if self.circumcircle is None:
return []
(xc, yc, r) = self.circumcircle
for triangle in self.neighbours:
if triangle is None:
continue
if triangle.circumcircle is None:
continue
(xc1, yc1, r1) = triangle.circumcircle
if (xc - xc1) ** 2 + (yc - yc1) ** 2 < r ** 2 + r1 ** 2:
p4 = [point for point in triangle.points if point not in self.points]
break
else:
return []
t1 = Triangle([p1, p2, p4])
t2 = Triangle([p2, p3, p4])
t3 = Triangle([p3, p1, p4])
t1.add_neighbours([t2, t3, self.neighbours[2]])
t2.add_neighbours([t3, t1, self.neighbours[0]])
t3.add_neighbours([t1, t2, self.neighbours[1]])
if self.neighbours[2] is not None:
self.neighbours[2].swap_neighbour(self, t1)
if self.neighbours[0] is not None:
self.neighbours[0].swap_neighbour(self, t2)
if self.neighbours[1] is not None:
self.neighbours[1].swap_neighbour(self, t3)
return [t1, t2, t3]
def add_neighbours(self, neighbours: List['Triangle']):
self.neighbours = neighbours
def swap_neighbour(self, old: 'Triangle', new: 'Triangle'):
if self.neighbours[0] == old:
self.neighbours[0] = new
elif self.neighbours[1] == old:
self.neighbours[1] = new
elif self.neighbours[2] == old:
self.neighbours[2] = new
def __eq__(self, other):
if isinstance(other, Triangle):
return set(self.points) == set(other.points)
return False
def __hash__(self):
return hash(tuple(sorted(self.points)))
class Delaunay:
def __init__(self, points: List[Tuple[float, float]]):
self.points = points
self.triangles = self.triangulate()
def triangulate(self):
x_min = min(self.points, key=lambda p: p[0])[0] - 1
y_min = min(self.points, key=lambda p: p[1])[1] - 1
x_max = max(self.points, key=lambda p: p[0])[0] + 1
y_max = max(self.points, key=lambda p: p[1])[1] + 1
super_triangle = Triangle([(x_min, y_min), (x_max, y_min), (x_min, y_max)])
triangles = [super_triangle]
for point in self.points:
bad_triangles = []
for triangle in triangles:
if triangle.contains(point):
bad_triangles.append(triangle)
polygon = []
for triangle in bad_triangles:
for p in triangle.points:
if all([p != tp for tp in point] for point in polygon):
polygon.append(p)
for triangle in bad_triangles:
triangles.remove(triangle)
new_triangles = []
for i in range(len(polygon)):
new_triangles.append(Triangle([polygon[i], polygon[(i + 1) % len(polygon)], point]))
for triangle in new_triangles:
for other_triangle in new_triangles:
if triangle == other_triangle:
continue
if set(triangle.points).intersection(set(other_triangle.points)):
triangle.add_neighbours([other_triangle if p in other_triangle.points else None for p in triangle.points])
for triangle in new_triangles:
triangles.append(triangle)
for triangle in triangles:
if any([p in super_triangle.points for p in triangle.points]):
continue
for i in range(3):
if triangle.neighbours[i] is None:
continue
if any([p in super_triangle.points for p in triangle.neighbours[i].points]):
continue
edge = {triangle.points[i], triangle.points[(i + 1) % 3]}
for j in range(3):
if triangle.neighbours[i].points[j] not in edge:
break
else:
triangle.neighbours[i] = None
triangles = [triangle for triangle in triangles if not any([p in super_triangle.points for p in triangle.points])]
return triangles
```
该实现中,Triangle类表示一个三角形,包含三个点和一个外接圆的信息。Delaunay类表示Delaunay三角剖分算法,包含一个点集和一个三角形集。在实现中,使用了超级三角形的概念,即将点集中所有点都插入到一个包含所有点的三角形中,然后再去除与超级三角形相邻的三角形。
阅读全文
相关推荐
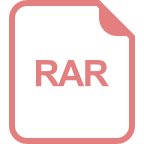














