写一个java自定义线程池分批导出excel代码
时间: 2023-06-04 07:06:21 浏览: 190
好的,我可以为您提供java自定义线程池分批导出excel的代码,以下是示例代码:
```java
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Font;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFCellStyle;
import org.apache.poi.xssf.usermodel.XSSFFont;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelExporter {
private static final int THREAD_COUNT = 4;
private static final int BATCH_SIZE = 2000;
private static ExecutorService threadPool =
Executors.newFixedThreadPool(THREAD_COUNT);
public void exportDataToExcel() throws Exception {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Data");
// Set header row style
Font headerFont = workbook.createFont();
headerFont.setBold(true);
headerFont.setColor(IndexedColors.WHITE.getIndex());
CellStyle headerCellStyle = workbook.createCellStyle();
headerCellStyle.setFont(headerFont);
headerCellStyle.setFillForegroundColor(IndexedColors.BLUE.getIndex());
headerCellStyle.setFillPattern(XSSFCellStyle.SOLID_FOREGROUND);
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("Column 1");
headerRow.createCell(1).setCellValue("Column 2");
headerRow.createCell(2).setCellValue("Column 3");
for (int i = 0; i < 3; i++) {
headerRow.getCell(i).setCellStyle(headerCellStyle);
}
// Start exporting data
for (int i = 0; i < getTotalRowCount(); i += BATCH_SIZE) {
int startRow = i + 1;
int endRow = Math.min(i + BATCH_SIZE, getTotalRowCount()) + 1;
threadPool.execute(
new ExportTask(
workbook,
sheet,
startRow,
endRow));
}
threadPool.shutdown();
threadPool.awaitTermination(Long.MAX_VALUE, TimeUnit.NANOSECONDS);
}
private static int getTotalRowCount() {
// Return the total number of rows to export
return 10000;
}
private static class ExportTask implements Runnable {
private final Workbook workbook;
private final Sheet sheet;
private final int startRow;
private final int endRow;
ExportTask(
Workbook workbook,
Sheet sheet,
int startRow,
int endRow) {
this.workbook = workbook;
this.sheet = sheet;
this.startRow = startRow;
this.endRow = endRow;
}
@Override
public void run() {
try {
// Simulate data retrieval
Thread.sleep(100);
// Export data to sheet
for (int i = startRow; i < endRow; i++) {
Row row = sheet.createRow(i);
row.createCell(0).setCellValue("Value " + i);
row.createCell(1).setCellValue("Value " + i * 2);
row.createCell(2).setCellValue("Value " + i * 3);
}
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
}
```
该代码中,我们使用了线程池来提高导出数据到excel时的效率。我们先设置一个每批次导出的行数,然后根据总行数和批次大小分批导出,每批次启动一个线程来处理。最后,等待所有线程完成后关闭线程池,导出工作完成。
阅读全文
相关推荐
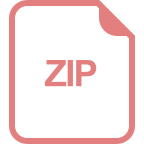
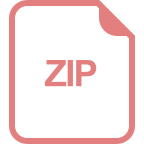
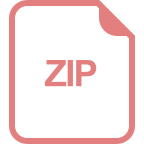
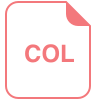
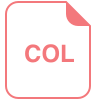
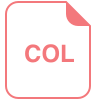
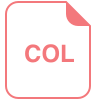
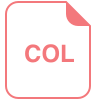
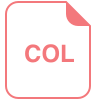
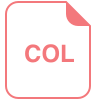
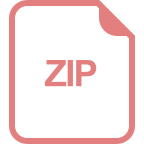
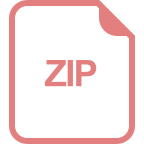
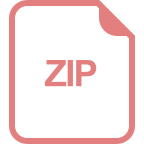
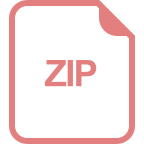