pthread_create详解
时间: 2023-09-09 22:06:11 浏览: 75
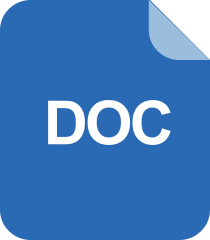
createthread函数用法详解
`pthread_create()` 是 POSIX 线程库中的函数,用于创建一个新线程,其使用方法如下:
```
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void *), void *arg);
```
函数参数解释如下:
- `thread`:指向线程标识符的指针。线程标识符是一个 `pthread_t` 类型的变量,用于唯一标识一个线程。
- `attr`:指向线程属性的指针。如果为 `NULL`,则使用默认线程属性。
- `start_routine`:线程启动时执行的函数。该函数的返回值为 `void *` 类型,参数为 `void *` 类型。
- `arg`:传递给 `start_routine` 函数的参数。
`pthread_create()` 成功返回 0,否则返回错误码。
以下是一个创建新线程的例子:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *print_message(void *arg)
{
char *message = (char *)arg;
printf("%s\n", message);
pthread_exit(NULL);
}
int main()
{
pthread_t thread_id;
char *message = "Hello, World!";
int ret = pthread_create(&thread_id, NULL, print_message, (void *)message);
if (ret != 0) {
printf("Failed to create thread.\n");
exit(EXIT_FAILURE);
}
pthread_join(thread_id, NULL);
return 0;
}
```
在上面的例子中,我们创建了一个新线程,该线程执行 `print_message()` 函数。该函数会打印传递给它的字符串参数,并退出线程。在主线程中,我们调用 `pthread_join()` 函数来等待新线程结束,并释放其资源。
注:`pthread_create()` 函数创建的线程是可执行的,即该线程会立即执行。如果需要控制线程的执行顺序,可以使用线程同步机制(如互斥锁、条件变量等)。
阅读全文
相关推荐
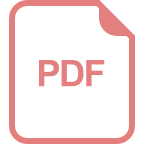
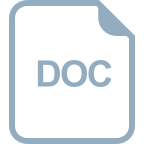
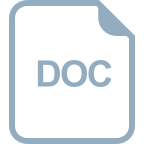
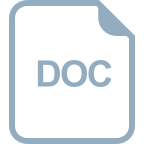












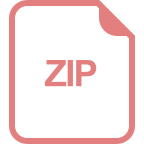