c语言将txt中的数据读到结构体链表中
时间: 2024-05-06 16:15:46 浏览: 120
假设txt文件中的每一行都是一个记录,包含了多个字段,我们可以定义一个结构体来存储每个记录的各个字段信息,然后使用链表把这些记录链接起来。
以下是一个示例代码,可以读取包含学生信息的txt文件,将其存储到一个链表中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 50
// 定义学生信息的结构体
typedef struct student {
char name[MAX_NAME_LEN];
int age;
int score;
struct student *next;
} Student;
// 从txt文件中读取学生信息并存储到链表中
void read_student_data(Student **head, const char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
fprintf(stderr, "Failed to open file %s\n", filename);
return;
}
char line[100];
while (fgets(line, sizeof(line), fp) != NULL) {
Student *new_student = (Student *)malloc(sizeof(Student));
if (new_student == NULL) {
fprintf(stderr, "Failed to allocate memory for new student\n");
break;
}
// 从txt文件中读取数据
sscanf(line, "%s %d %d", new_student->name, &new_student->age, &new_student->score);
// 将新的学生信息添加到链表中
new_student->next = *head;
*head = new_student;
}
fclose(fp);
}
// 打印链表中的学生信息
void print_student_data(const Student *head) {
printf("Name\tAge\tScore\n");
const Student *p = head;
while (p != NULL) {
printf("%s\t%d\t%d\n", p->name, p->age, p->score);
p = p->next;
}
}
int main() {
Student *head = NULL;
read_student_data(&head, "students.txt");
print_student_data(head);
return 0;
}
```
在这个示例代码中,我们定义了一个 `Student` 结构体来存储每个记录的各个字段信息。在 `read_student_data` 函数中,我们打开指定的txt文件,逐行读取文件内容,并使用 `sscanf` 函数从每行数据中提取学生的姓名、年龄和分数等信息,并将这些信息存储到一个新的 `Student` 结构体中。然后,我们将新的学生信息添加到链表头部,最终得到一个包含所有学生信息的链表。在 `print_student_data` 函数中,我们遍历链表并打印每个学生的信息。
阅读全文
相关推荐
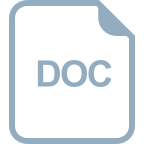
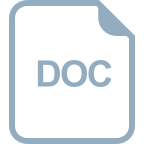
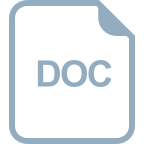
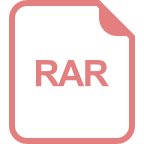
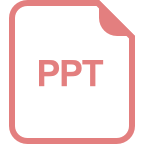
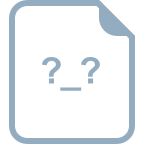
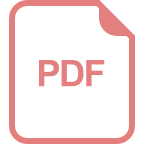
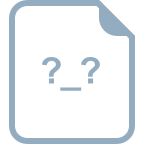
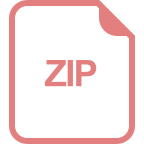
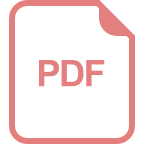
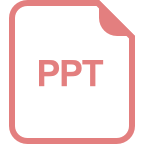
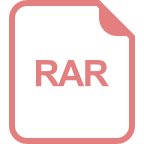
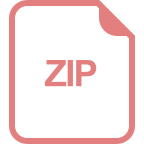
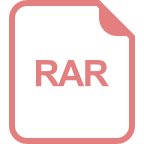
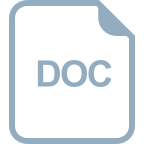
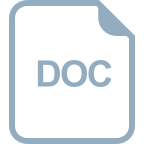