用java创建一个功能完好的记事本程序,包括增, 删 ,改 ,查
时间: 2023-05-23 18:07:32 浏览: 106
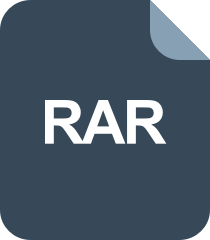
Java写的记事本程序功能完整
答:好的,下面是用Java创建一个基本的记事本程序,包括增,删,改,查的实现方式:
1. 创建一个Java项目,命名为“NotePad”,在该项目中创建一个Java类,命名为“Note”;
2. 在“Note”类中,定义一个ArrayList集合,用于存储记事本中的所有内容;
3. 定义一个无参数的构造方法,用于初始化ArrayList集合;
4. 定义一个“addNote”方法,用于向ArrayList集合中添加新的笔记;
```java
public void addNote(String note){
notes.add(note);
System.out.println("笔记添加成功!");
}
```
5. 定义一个“delNote”方法,用于从ArrayList集合中删除某个笔记;
```java
public void delNote(int index){
if(index >= 0 && index < notes.size()){
notes.remove(index);
System.out.println("笔记删除成功!");
}else{
System.out.println("没有找到要删除的笔记!");
}
}
```
6. 定义一个“updateNote”方法,用于修改ArrayList集合中的某个笔记;
```java
public void updateNote(int index, String note){
if(index >= 0 && index < notes.size()){
notes.set(index, note);
System.out.println("笔记修改成功!");
}else{
System.out.println("没有找到要修改的笔记!");
}
}
```
7. 定义一个“searchNote”方法,用于根据关键字在ArrayList集合中查找相应的笔记;
```java
public void searchNote(String keyword){
boolean hasResult = false;
for(String note : notes){
if(note.contains(keyword)){
System.out.println(note);
hasResult = true;
}
}
if(!hasResult){
System.out.println("没有找到相关的笔记!");
}
}
```
8. 在主函数中,创建一个Note对象,然后测试addNote、delNote、updateNote、searchNote方法的功能。
下面是完整的Note类代码:
```java
import java.util.ArrayList;
public class Note {
private ArrayList<String> notes;
public Note() {
notes = new ArrayList<String>();
}
public void addNote(String note){
notes.add(note);
System.out.println("笔记添加成功!");
}
public void delNote(int index){
if(index >= 0 && index < notes.size()){
notes.remove(index);
System.out.println("笔记删除成功!");
}else{
System.out.println("没有找到要删除的笔记!");
}
}
public void updateNote(int index, String note){
if(index >= 0 && index < notes.size()){
notes.set(index, note);
System.out.println("笔记修改成功!");
}else{
System.out.println("没有找到要修改的笔记!");
}
}
public void searchNote(String keyword){
boolean hasResult = false;
for(String note : notes){
if(note.contains(keyword)){
System.out.println(note);
hasResult = true;
}
}
if(!hasResult){
System.out.println("没有找到相关的笔记!");
}
}
}
```
通过调用该类中的四个方法,就可以实现记事本的完整功能。
阅读全文
相关推荐
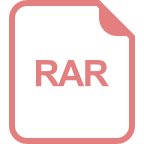





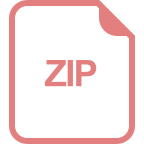



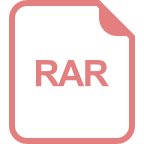
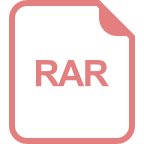
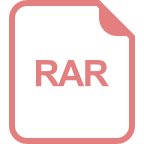



