opencv四个顶点画四边形
时间: 2024-05-27 18:07:22 浏览: 29
要画一个四边形,可以使用 OpenCV 中的 `cv2.line()` 函数画四条直线来实现,最后将第一条直线的起点和最后一条直线的终点相连就可以了。
以下是用 Python 代码实现的示例:
```
import cv2
import numpy as np
# 创建一个黑色的图像
img = np.zeros((512,512,3), np.uint8)
# 定义四个顶点坐标
pts = np.array([[10,50], [200,50], [200,100], [10,100]], np.int32)
# 在图像上画出四条边
cv2.line(img, (pts,pts), (pts,pts), (0,255,0), 2)
cv2.line(img, (pts,pts), (pts,pts), (0,255,0), 2)
cv2.line(img, (pts,pts), (pts,pts), (0,255,0), 2)
cv2.line(img, (pts,pts), (pts,pts), (0,255,0), 2)
# 显示图像
cv2.imshow("image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关问题
python opencv 识别四边形的四个顶点
使用Python和OpenCV可以轻松地识别四边形的四个顶点。以下是一个基本的方法:
1. 导入所需的库:
```python
import cv2
import numpy as np
```
2. 读取图像并进行预处理:
```python
image = cv2.imread('image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150)
```
3. 在图像中检测轮廓:
```python
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
4. 对检测到的轮廓进行筛选,只保留面积最大的轮廓:
```python
contour = max(contours, key=cv2.contourArea)
```
5. 对轮廓进行逼近,以减少顶点的数量:
```python
epsilon = 0.04 * cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, epsilon, True)
```
6. 绘制顶点的位置:
```python
for point in approx:
cv2.circle(image, tuple(point[0]), 5, (0, 255, 0), -1)
```
7. 显示结果:
```python
cv2.imshow('Result', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样,你就可以使用Python和OpenCV识别图像中四边形的四个顶点了。请将代码中的'image.jpg'替换为你要识别的图像路径。运行代码后,你将在窗口中看到标记有四个顶点的图像。
opencv 截取不规则四边形 c++
OpenCV是一个开源的计算机视觉库,提供了丰富的图像处理和计算机视觉算法。它支持多种编程语言,包括C++、Python等。在C++中,你可以使用OpenCV来截取不规则四边形的图像。
要截取不规则四边形,你可以按照以下步骤进行操作:
1. 定义四边形的四个顶点坐标。
2. 创建一个与原始图像大小相同的空白图像。
3. 使用OpenCV的函数`cv::fillConvexPoly`将四边形区域填充为白色。
4. 使用OpenCV的函数`cv::bitwise_and`将原始图像与填充后的图像进行按位与操作,得到截取后的图像。
下面是一个示例代码:
```cpp
#include <opencv2/opencv.hpp>
int main()
{
// 读取原始图像
cv::Mat image = cv::imread("input.jpg");
// 定义四边形的四个顶点坐标
std::vector<cv::Point> vertices;
vertices.push_back(cv::Point(100, 100));
vertices.push_back(cv::Point(200, 150));
vertices.push_back(cv::Point(300, 200));
vertices.push_back(cv::Point(200, 250));
// 创建空白图像
cv::Mat mask = cv::Mat::zeros(image.size(), CV_8UC1);
// 填充四边形区域
cv::fillConvexPoly(mask, vertices.data(), vertices.size(), cv::Scalar(255));
// 按位与操作
cv::Mat result;
cv::bitwise_and(image, mask, result);
// 显示结果图像
cv::imshow("Result", result);
cv::waitKey(0);
return 0;
}
```
这段代码会读取名为"input.jpg"的原始图像,然后根据定义的四边形顶点坐标截取不规则四边形的图像,并显示结果。
相关推荐
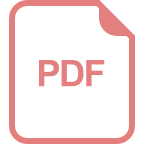












