使用Java编写牛顿均差型插值多项式的构造,要求可以输入数表,可以输入使用几次插值计算,可以输入计算的自变量f的值。要完整代码
时间: 2024-12-23 17:16:18 浏览: 6
在Java中,我们可以创建一个牛顿插值多项式(也称为拉格朗日插值)的类,该类允许用户输入数表、插值次数以及计算的自变量值。下面是一个简单的示例代码,它实现了这个功能:
```java
import java.util.Scanner;
public class NewtonInterpolator {
private double[][] coefficients; // 存储多项式的系数
public NewtonInterpolator(double[] xValues, double[] yValues) {
if (xValues.length != yValues.length + 1) {
throw new IllegalArgumentException("Number of x and y values must be the same or differ by one.");
}
coefficients = new double[yValues.length][];
for (int i = 0; i < yValues.length; i++) {
coefficients[i] = new double[i + 1];
for (int j = 0; j <= i; j++) {
coefficients[i][j] = yValues[i - j] / fact(i - j);
for (int k = 0; k < j; k++) {
coefficients[i][j] *= xValues[i - k];
}
}
}
}
private double fact(int n) {
if (n == 0 || n == 1) return 1;
else return n * fact(n - 1);
}
public double interpolate(double f) {
int degree = coefficients[coefficients.length - 1].length - 1;
double result = coefficients[0][degree];
for (int i = 1; i <= degree; i++) {
result += coefficients[i][degree] * Math.pow(f, degree - i);
}
return result;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter number of data points (including end points): ");
int numPoints = scanner.nextInt();
double[] xValues = new double[numPoints];
double[] yValues = new double[numPoints];
System.out.println("Enter x values: ");
for (int i = 0; i < numPoints; i++) {
xValues[i] = scanner.nextDouble();
}
System.out.println("Enter y values: ");
for (int i = 0; i < numPoints; i++) {
yValues[i] = scanner.nextDouble();
}
System.out.println("Enter interpolation order: ");
int interpolationOrder = scanner.nextInt();
NewtonInterpolator interpolator = new NewtonInterpolator(xValues, yValues);
System.out.print("Enter a value for f to interpolate: ");
double f = scanner.nextDouble();
double interpolatedValue = interpolator.interpolate(f);
System.out.printf("Interpolated value at %f is %f\n", f, interpolatedValue);
scanner.close();
}
}
```
在这个例子中,`NewtonInterpolator`类接受两个数组作为输入,然后根据给定的插值次数计算并存储牛顿插值多项式的系数。`interpolate`方法用于实际的插值计算。
请注意,这只是一个基本实现,实际应用中可能会需要更多的错误处理和优化。运行此程序时,用户会被提示输入数据点的数量、坐标值以及插值的次数。然后用户可以输入一个自变量`f`,程序会返回对应的数据点处的插值结果。
阅读全文
相关推荐
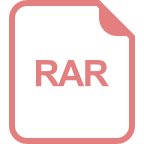
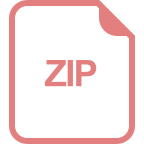





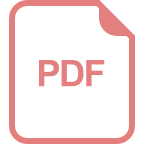
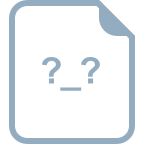
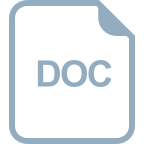


