用java代码 编写关于牛顿均差型插值多项式的构造
时间: 2024-12-21 15:30:22 浏览: 6
在Java中,我们可以使用泰勒级数的思想来编写牛顿均差型插值多项式。牛顿均差型插值是一种基于数据点构建多项式函数的方法,用于近似连续函数。以下是简单的步骤和代码示例:
```java
public class NewtonInterpolation {
private double[] xValues; // 数据点的x坐标
private double[] yValues; // 对应的数据点y坐标
public NewtonInterpolation(double[] x, double[] y) {
this.xValues = x;
this.yValues = y;
}
// 计算均差型插值系数
private double[] computeCoefficients() {
int n = xValues.length - 1;
double[] coefficients = new double[n + 1];
for (int i = n; i >= 0; i--) {
double sum = 0;
for (int j = 0; j <= i; j++) {
if (j != i) {
sum += yValues[j] / factorial(i - j);
}
}
coefficients[i] = yValues[n] / factorial(n - i) - sum;
}
return coefficients;
}
// 计算阶乘
private static long factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
// 构造插值多项式并返回其表达式
public String interpolatePolynomialString() {
StringBuilder polynomial = new StringBuilder();
double[] coefficients = computeCoefficients();
for (int i = 0; i <= coefficients.length - 1; i++) {
polynomial.append(coefficients[i]);
if (i != coefficients.length - 1) {
polynomial.append(" * (x - ").append(xValues[i]).append(")");
}
if (i > 0) {
polynomial.append(" + ");
}
}
// 移除最后一个加号
polynomial.deleteCharAt(polynomial.length() - 1);
return polynomial.toString();
}
public static void main(String[] args) {
double[] xData = {0, 1, 2, 3};
double[] yData = {1, 4, 9, 16};
NewtonInterpolation interpolator = new NewtonInterpolation(xData, yData);
System.out.println("牛顿均差型插值多项式: " + interpolator.interpolatePolynomialString());
}
}
```
这个例子中,`NewtonInterpolation`类接收两个数组(数据点的x和y坐标),计算均差型插值系数,并生成相应的插值多项式字符串。注意这只是一个基础实现,实际应用中可能需要处理边界条件和异常。
阅读全文
相关推荐
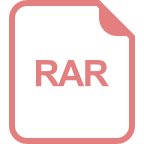
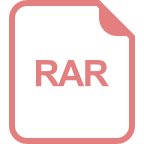
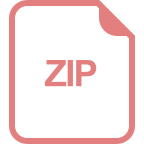















