写一个程序,定义抽象基类Container,由它派生出三个派生类:Sphere(球体)、Cylinder(圆柱体)、Cube(正方体)。用虚函数分别计算几种图形的表面积和体积。
时间: 2024-02-11 07:08:15 浏览: 152
好的,以下是您所需的程序:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 定义抽象基类 Container
class Container {
public:
// 定义纯虚函数计算表面积
virtual double surface_area() = 0;
// 定义纯虚函数计算体积
virtual double volume() = 0;
};
// 派生类 Sphere(球体)
class Sphere : public Container {
private:
double radius;
public:
Sphere(double r) {
radius = r;
}
// 实现计算表面积的虚函数
double surface_area() {
return 4 * M_PI * radius * radius;
}
// 实现计算体积的虚函数
double volume() {
return 4 * M_PI * radius * radius * radius / 3;
}
};
// 派生类 Cylinder(圆柱体)
class Cylinder : public Container {
private:
double radius;
double height;
public:
Cylinder(double r, double h) {
radius = r;
height = h;
}
// 实现计算表面积的虚函数
double surface_area() {
return 2 * M_PI * radius * height + 2 * M_PI * radius * radius;
}
// 实现计算体积的虚函数
double volume() {
return M_PI * radius * radius * height;
}
};
// 派生类 Cube(正方体)
class Cube : public Container {
private:
double length;
public:
Cube(double l) {
length = l;
}
// 实现计算表面积的虚函数
double surface_area() {
return 6 * length * length;
}
// 实现计算体积的虚函数
double volume() {
return length * length * length;
}
};
int main() {
// 创建不同的图形对象
Sphere sphere(5);
Cylinder cylinder(3, 10);
Cube cube(4);
// 通过指针调用虚函数计算表面积和体积
Container *p = &sphere;
cout << "Sphere surface area: " << p->surface_area() << endl;
cout << "Sphere volume: " << p->volume() << endl;
p = &cylinder;
cout << "Cylinder surface area: " << p->surface_area() << endl;
cout << "Cylinder volume: " << p->volume() << endl;
p = &cube;
cout << "Cube surface area: " << p->surface_area() << endl;
cout << "Cube volume: " << p->volume() << endl;
return 0;
}
```
程序中我们定义了一个抽象基类 Container,它包含两个纯虚函数 surface_area 和 volume,用于计算图形的表面积和体积。然后我们派生出了三个派生类 Sphere、Cylinder 和 Cube,分别表示球体、圆柱体和正方体,并重写了抽象基类中的两个纯虚函数。最后在主函数中,我们创建了不同的图形对象,并通过指针调用虚函数计算表面积和体积。
阅读全文
相关推荐
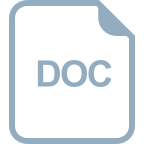
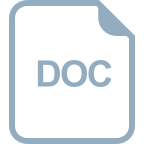
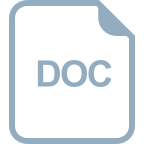







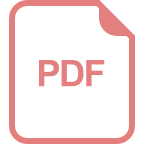
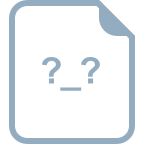
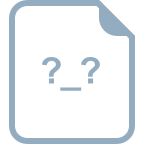
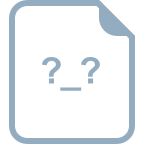
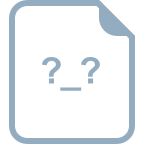
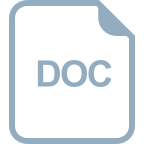
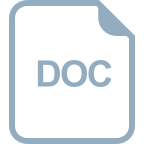

