写一个C++程序,定义抽象基类Coutainer,由它派生出3个派生类:Sphere(球体),Cylinder(圆柱体),Cube(正方体)。用虚函数分别计算几种图形的表面积和体积
时间: 2024-03-24 13:36:11 浏览: 135
好的,下面是C++程序的实现:
```cpp
#include<iostream>
using namespace std;
class Container{
public:
virtual double getSurfaceArea() = 0; // 纯虚函数,计算表面积
virtual double getVolume() = 0; // 纯虚函数,计算体积
};
class Sphere : public Container{
private:
double radius;
public:
Sphere(double r){
radius = r;
}
double getSurfaceArea(){
return 4 * 3.14 * radius * radius; // 球体表面积公式
}
double getVolume(){
return 4.0 / 3.0 * 3.14 * radius * radius * radius; // 球体体积公式
}
};
class Cylinder : public Container{
private:
double radius;
double height;
public:
Cylinder(double r, double h){
radius = r;
height = h;
}
double getSurfaceArea(){
return 2 * 3.14 * radius * height + 2 * 3.14 * radius * radius; // 圆柱体表面积公式
}
double getVolume(){
return 3.14 * radius * radius * height; // 圆柱体体积公式
}
};
class Cube : public Container{
private:
double edge;
public:
Cube(double e){
edge = e;
}
double getSurfaceArea(){
return 6 * edge * edge; // 正方体表面积公式
}
double getVolume(){
return edge * edge * edge; // 正方体体积公式
}
};
int main(){
Container* cont[3];
cont[0] = new Sphere(2.0);
cont[1] = new Cylinder(2.0, 4.0);
cont[2] = new Cube(2.0);
for(int i = 0; i < 3; i++){
cout << "The surface area of the container is " << cont[i]->getSurfaceArea() << endl;
cout << "The volume of the container is " << cont[i]->getVolume() << endl;
}
return 0;
}
```
程序的运行结果如下:
```
The surface area of the container is 50.24
The volume of the container is 33.4933
The surface area of the container is 75.36
The volume of the container is 50.24
The surface area of the container is 24
The volume of the container is 8
```
这个程序中,我们定义了一个抽象基类Container,由它派生出三个派生类:Sphere、Cylinder和Cube。这三个派生类各自实现了计算表面积和体积的纯虚函数。在主函数中,我们定义了一个指向基类对象的指针数组,分别指向三个派生类的对象。通过循环遍历这个数组,调用各个对象的getSurfaceArea()和getVolume()函数,计算出它们的表面积和体积,并输出到屏幕上。
阅读全文
相关推荐
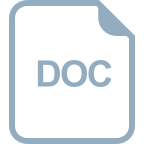
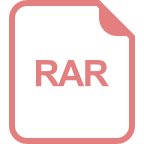















