c++使用虚函数求球体和圆柱体的体积和表面积
时间: 2023-09-29 20:02:58 浏览: 90
以下是使用虚函数求球体和圆柱体的体积和表面积的C++代码示例:
```
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
public:
virtual double getVolume() = 0;
virtual double getSurfaceArea() = 0;
};
class Sphere : public Shape {
private:
double radius;
public:
Sphere(double r) {
radius = r;
}
double getVolume() {
return (4.0/3.0) * M_PI * pow(radius, 3);
}
double getSurfaceArea() {
return 4 * M_PI * pow(radius, 2);
}
};
class Cylinder : public Shape {
private:
double radius;
double height;
public:
Cylinder(double r, double h) {
radius = r;
height = h;
}
double getVolume() {
return M_PI * pow(radius, 2) * height;
}
double getSurfaceArea() {
return 2 * M_PI * radius * (radius + height);
}
};
int main() {
Shape* shape;
Sphere sphere(2.0);
Cylinder cylinder(2.0, 5.0);
shape = &sphere;
cout << "Sphere Volume: " << shape->getVolume() << endl;
cout << "Sphere Surface Area: " << shape->getSurfaceArea() << endl;
shape = &cylinder;
cout << "Cylinder Volume: " << shape->getVolume() << endl;
cout << "Cylinder Surface Area: " << shape->getSurfaceArea() << endl;
return 0;
}
```
在上面的代码中,我们定义了一个抽象基类`Shape`,包含两个纯虚函数`getVolume`和`getSurfaceArea`。然后,我们定义了两个派生类`Sphere`和`Cylinder`,分别表示球体和圆柱体。这两个派生类继承了`Shape`类,并实现了`getVolume`和`getSurfaceArea`函数。
在`main`函数中,我们首先创建了一个`Sphere`对象和一个`Cylinder`对象,并将它们的地址分别赋给一个`Shape`指针变量`shape`。然后,我们通过`shape`指针调用了`getVolume`和`getSurfaceArea`函数,分别输出了球体和圆柱体的体积和表面积。
需要注意的是,我们在`Shape`类中将`getVolume`和`getSurfaceArea`函数声明为纯虚函数,这意味着我们不能直接创建`Shape`对象,而只能通过派生类来创建对象。这种技术称为抽象类,它可以帮助我们实现多态性,即在运行时根据对象的实际类型来调用相应的函数。
相关推荐
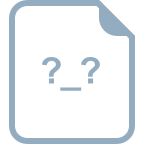















