设计一个继承家族的类,点派生出圆类,以圆类为基类派生出圆柱体类和球体类c++
时间: 2024-12-01 10:10:29 浏览: 23
在 C++ 中,你可以设计一个简单的类层次结构来表示几何形状,比如圆形、圆柱体和球体。首先,我们创建一个基础类 `Shape`,然后让 `Circle`, `Cylinder`, 和 `Sphere` 继承自这个基础类。
```cpp
#include <iostream>
#include <cmath>
// 基础形状类 Shape
class Shape {
public:
// 构造函数
Shape(double radius) : radius(radius) {}
// 简单的属性和操作
virtual double getArea() const = 0; // 静态纯虚函数,用于计算面积
virtual double getVolume() const = 0; // 静态纯虚函数,用于计算体积
protected:
double radius;
};
// 圆形类 Circle 继承自 Shape
class Circle : public Shape {
private:
double circumference;
public:
Circle(double r) : Shape(r), circumference(2 * M_PI * r) {} // 计算周长并存储
double getArea() const override { return M_PI * pow(radius, 2); }
double getVolume() const override { return 0; } // 因为圆没有体积,返回0
};
// 圆柱体类 Cylinder 继承自 Shape
class Cylinder : public Shape {
private:
double height;
public:
Cylinder(double r, double h) : Shape(r), height(h) {}
double getArea() const override { return M_PI * pow(radius, 2) + 2 * M_PI * radius * height; }
double getVolume() const override { return M_PI * pow(radius, 2) * height; }
};
// 球体类 Sphere 继承自 Shape
class Sphere : public Shape {
private:
double diameter;
public:
Sphere(double d) : Shape(d / 2), diameter(d) {}
double getArea() const override { return 4 * M_PI * pow(radius, 2); }
double getVolume() const override { return (4 / 3) * M_PI * pow(radius, 3); }
};
int main() {
Circle circle(5);
std::cout << "Circle area: " << circle.getArea() << ", volume: " << circle.getVolume() << "\n";
Cylinder cylinder(3, 7);
std::cout << "Cylinder area: " << cylinder.getArea() << ", volume: " << cylinder.getVolume() << "\n";
Sphere sphere(10);
std::cout << "Sphere area: " << sphere.getArea() << ", volume: " << sphere.getVolume() << "\n";
return 0;
}
```
在这个例子中,`Shape` 是一个抽象基类,它定义了共同的行为(如计算面积和体积)。`Circle`, `Cylinder`, 和 `Sphere` 分别继承并实现了各自特有的属性和计算方法。
阅读全文
相关推荐
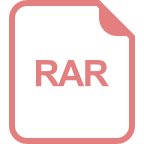
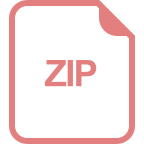
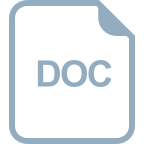
















