如何利用window.onresize来监听元素大小的变化
时间: 2024-09-13 07:16:16 浏览: 102
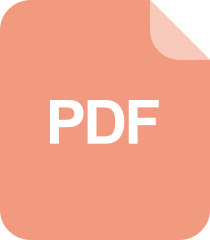
使用jQuery监听DOM元素大小变化
`window.onresize` 是 JavaScript 中的一个全局事件处理器,用于监听窗口大小调整时触发的事件。如果你想监听某个特定元素的大小变化,而不是整个窗口,你不能直接使用 `onresize`,因为它的目标是整个视口。但是,你可以结合其他技术来达到目的:
1. **监测元素位置**:
使用 `getBoundingClientRect()` 方法,可以获取元素相对于视窗的位置信息,包括宽度和高度。你可以定期检查元素的尺寸变化,例如每隔一定时间:
```javascript
function checkElementSize(element) {
var rect = element.getBoundingClientRect();
var currentWidth = rect.width;
var currentHeight = rect.height;
// 如果尺寸有变化
if (currentWidth !== oldWidth || currentHeight !== oldHeight) {
// 更新尺寸变量并处理变化
oldWidth = currentWidth;
oldHeight = currentHeight;
console.log('Element resized:', { width: currentWidth, height: currentHeight });
}
// 触发周期性的检查,例如每秒一次
setTimeout(() => checkElementSize(element), 1000);
}
// 初始化尺寸记录
var oldWidth = element.offsetWidth;
var oldHeight = element.offsetHeight;
// 开始监听
checkElementSize(element);
```
2. **MutationObserver**:
如果允许使用新特性,你可以使用 MutationObserver 来监听元素的尺寸变化,尽管它主要是为DOM结构更改设计的:
```javascript
const observer = new MutationObserver((mutationsList) => {
mutationsList.forEach((mutation) => {
if (mutation.type === 'attributes' && ['width', 'height'].includes(mutation.attributeName)) {
// 处理尺寸变化
console.log('Element resized:', element.style[mutation.attributeName]);
}
});
});
observer.observe(element, { attributes: true });
```
这两种方法都有其局限性,比如定时检查可能导致性能开销,而 MutationObserver 则受限于旧版浏览器的支持。在实际应用中,应考虑你的目标浏览器环境,以及对性能的要求。
阅读全文
相关推荐
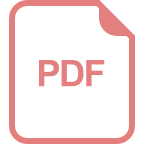
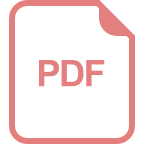















