c++统计不重复的单词个数
时间: 2023-11-13 15:02:01 浏览: 53
你可以使用哈希表来统计不重复的单词个数。具体实现方法如下:
1. 将文本按照空格和标点符号分割成单词,可以使用 strtok 函数实现。
2. 对于每个单词,将它加入哈希表中。如果该单词已经存在于哈希表中,则不做任何操作。
3. 统计哈希表中不同单词的数量。
下面是一个示例代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define TABLE_SIZE 10007
typedef struct Node {
char *key;
struct Node *next;
} Node;
int hash(const char *s) {
int h = 0;
while (*s) {
h = (h * 131 + *s++) % TABLE_SIZE;
}
return h;
}
void insert(Node **table, const char *key) {
int h = hash(key);
Node *p = table[h];
while (p) {
if (strcmp(p->key, key) == 0) {
return;
}
p = p->next;
}
p = malloc(sizeof(Node));
p->key = strdup(key);
p->next = table[h];
table[h] = p;
}
int count(Node **table) {
int cnt = 0;
for (int i = 0; i < TABLE_SIZE; i++) {
Node *p = table[i];
while (p) {
cnt++;
p = p->next;
}
}
return cnt;
}
void destroy(Node **table) {
for (int i = 0; i < TABLE_SIZE; i++) {
Node *p = table[i];
while (p) {
Node *q = p;
p = p->next;
free(q->key);
free(q);
}
}
free(table);
}
int main() {
char text[] = "Hello, world! This is a test. Hello, world! 123";
char *p = strtok(text, " ,.!?");
Node **table = calloc(TABLE_SIZE, sizeof(Node *));
while (p) {
insert(table, p);
p = strtok(NULL, " ,.!?");
}
int cnt = count(table);
printf("The number of different words is %d.\n", cnt);
destroy(table);
return 0;
}
```
该程序的时间复杂度为 $O(N)$,其中 $N$ 是单词的总数。如果哈希表的大小适当调整,其空间复杂度也可以达到 $O(N)$ 级别。
相关推荐
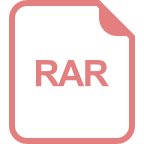














