线性回归的梯度下降法用python代码实现求解波士顿房价
时间: 2023-06-04 10:05:18 浏览: 66
以下是Python代码实现:
```
# 导入所需库
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 读入数据
data = pd.read_csv('boston.csv')
X = data.iloc[:, :-1]
y = data.iloc[:, -1]
m = len(y)
# 特征归一化
X = (X - np.mean(X)) / np.std(X)
# 添加一列全为1的特征值
X['ones'] = np.ones(m)
# 初始化参数向量theta
theta = np.zeros(len(X.columns))
# 定义代价函数并初始化
def costfunction(X, y, theta):
J = np.sum(np.power(np.dot(X, theta) - y, 2)) / (2 * m)
return J
J = costfunction(X, y, theta)
# 定义梯度下降函数
def gradientdescent(X, y, theta, alpha, num_iters):
J_history = np.zeros(num_iters)
for i in range(num_iters):
theta = theta - alpha * np.dot(X.T, np.dot(X, theta) - y) / m
J_history[i] = costfunction(X, y, theta)
return theta, J_history
# 进行梯度下降求解
alpha = 0.01
num_iters = 1000
theta, J_history = gradientdescent(X, y, theta, alpha, num_iters)
# 输出训练后得到的最优参数
print('最优参数为:', theta)
# 绘制代价函数迭代曲线
plt.plot(range(num_iters), J_history)
plt.xlabel('迭代次数')
plt.ylabel('代价函数J值')
plt.show()
```
以上就是用Python代码实现线性回归的梯度下降法求解波士顿房价的过程。
相关推荐
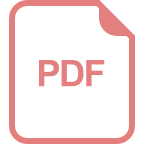
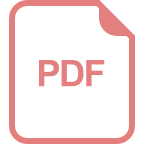
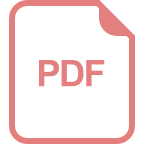














