解释代码含义“Panels: - Class: rviz/Displays Help Height: 0 Name: Displays Property Tree Widget: Expanded: - /Global Options1 - /Status1 Splitter Ratio: 0.5 Tree Height: 627 - Class: rviz/Selection Name: Selection - Class: rviz/Tool Properties Expanded: - /2D Pose Estimate1 - /2D Nav Goal1 - /Publish Point1 Name: Tool Properties Splitter Ratio: 0.5886790156364441 - Class: rviz/Views Expanded: - /Current View1 Name: Views Splitter Ratio: 0.5 - Class: rviz/Time Experimental: false Name: Time SyncMode: 0 SyncSource: "" Preferences: PromptSaveOnExit: true Toolbars: toolButtonStyle: 2”
时间: 2024-02-10 12:11:11 浏览: 127
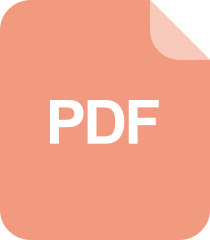
机器嗅觉的气味表征和繁殖:综述
这段代码是一份RViz配置文件,用于配置RViz(机器人可视化工具)中的各种面板(Panels)、首选项(Preferences)和工具栏(Toolbars)。具体解释如下:
- Panels:定义了四个面板,分别是Displays、Selection、Tool Properties和Views。每个面板都有一个Class属性,用于指定该面板使用的RViz插件类型。同时,还可以定义面板的名称、高度、展开状态、分割比例等属性。
- Preferences:定义了一个PromptSaveOnExit属性,用于控制RViz在退出时是否提示用户保存配置。
- Toolbars:定义了一个toolButtonStyle属性,用于指定RViz工具栏按钮的样式。
这份配置文件可以通过RViz的GUI界面进行编辑和保存,用于定制RViz的外观和功能。
阅读全文
相关推荐
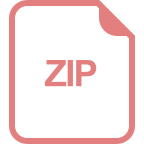
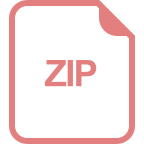
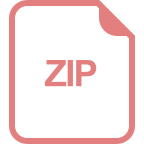
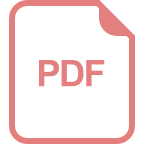
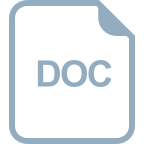
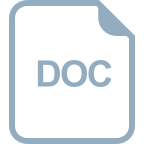










