Write a program to display font sizes Layout It should have: ● In the top: - label for letter “X” - label for font size value ● In the bottom: - button to increase font size - button to decrease font size Desired final look: Functionality Initially, the X label should display an 18 point “X”, and the font size label should display the value “18”. Every time the “increase” button is selected, the font size of the “X” and the value in the font size label should be increased by 1. Every time the “decrease” button is selected, the font size of the “X” and the value in the font size label should be decreased by 1. Make sure that this does not allow negative font sizes.
时间: 2024-01-14 10:02:38 浏览: 61
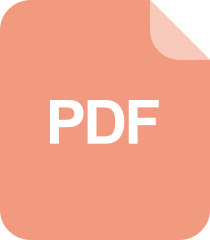
标准C++类string的Copy-On-Write技术
Here is an example program written in Java using Swing library to display font sizes:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class FontSizeDisplay extends JFrame {
private JLabel xLabel;
private JLabel fontSizeLabel;
private int fontSize = 18;
public FontSizeDisplay() {
setTitle("Font Size Display");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 200);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
// Top panel with X label and font size label
JPanel topPanel = new JPanel(new GridLayout(2, 1));
xLabel = new JLabel("X", SwingConstants.CENTER);
xLabel.setFont(new Font("Arial", Font.PLAIN, fontSize));
fontSizeLabel = new JLabel(Integer.toString(fontSize), SwingConstants.CENTER);
topPanel.add(xLabel);
topPanel.add(fontSizeLabel);
add(topPanel, BorderLayout.CENTER);
// Bottom panel with increase and decrease buttons
JPanel bottomPanel = new JPanel(new GridLayout(1, 2));
JButton increaseButton = new JButton("Increase");
increaseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
fontSize++;
if (fontSize > 0) {
xLabel.setFont(new Font("Arial", Font.PLAIN, fontSize));
fontSizeLabel.setText(Integer.toString(fontSize));
} else {
fontSize--;
}
}
});
JButton decreaseButton = new JButton("Decrease");
decreaseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
fontSize--;
if (fontSize > 0) {
xLabel.setFont(new Font("Arial", Font.PLAIN, fontSize));
fontSizeLabel.setText(Integer.toString(fontSize));
} else {
fontSize++;
}
}
});
bottomPanel.add(increaseButton);
bottomPanel.add(decreaseButton);
add(bottomPanel, BorderLayout.SOUTH);
}
public static void main(String[] args) {
FontSizeDisplay app = new FontSizeDisplay();
app.setVisible(true);
}
}
```
In this program, we create a JFrame window with a BorderLayout layout. In the center of the window, we create a top panel with X label and font size label, and a bottom panel with increase and decrease buttons. The X label is initially set to font size 18, and the font size label displays the value "18".
The increase and decrease buttons have action listeners that update the font size of the X label and the value in the font size label. We also make sure that the font size does not become negative.
When you run this program, you should see a window with an X label and a font size label in the center, and increase and decrease buttons at the bottom. Clicking the increase button will increase the font size of the X label and the value in the font size label by 1, and clicking the decrease button will decrease the font size by 1.
阅读全文
相关推荐
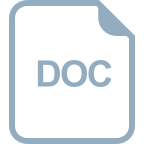
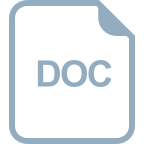

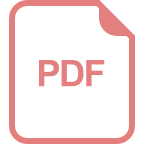
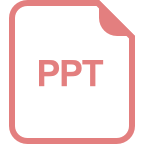
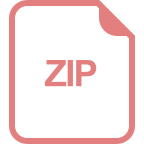
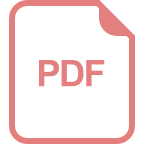
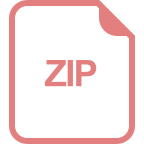
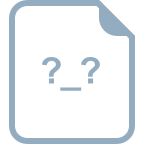
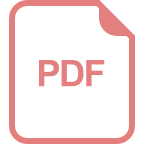
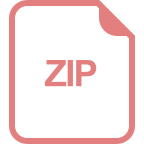
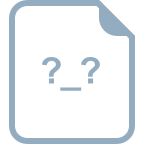
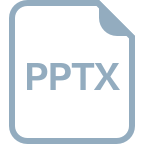
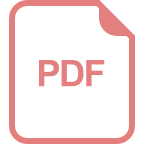
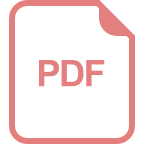
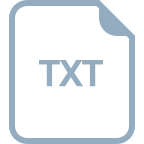
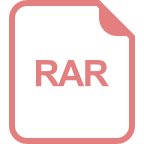
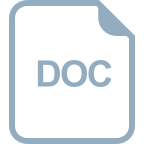