(vector<int>& weights) 是什么
时间: 2023-12-03 11:04:23 浏览: 54
`(vector<int>& weights)` 是一个函数参数,表示一个整数向量的引用。在C++中,使用&符号可以将一个变量声明为引用,这意味着它将成为原始变量的别名,而不是一个新的独立变量。在这个函数中,使用引用作为参数可以避免在函数调用时进行复制操作,从而提高了程序的效率。
具体来说,`(vector<int>& weights)` 表示一个整数向量的引用,该向量存储了每个字符的出现频率。在Huffman编码算法中,首先需要计算每个字符的出现频率,然后使用这些频率构建Huffman编码树。因此,将这个向量作为函数参数传递可以方便地将字符的频率传递给Huffman编码树的构建函数。
相关问题
HuffmanNode* build_huffman_tree(vector<int>& weights)是什么
`HuffmanNode* build_huffman_tree(vector<int>& weights)` 是一个函数,用于构建Huffman编码树。它接受一个表示字符频率的整数向量weights作为输入参数,并返回一个指向HuffmanNode类型对象的指针,该节点表示整个Huffman编码树的根节点。该函数使用了优先队列来实现Huffman树的构建过程,具体流程如下:
1. 首先,将每个字符的出现频率表示为一个HuffmanNode对象,并将其插入到一个优先队列中。这里使用的是STL库中的priority_queue。
2. 然后,从优先队列中取出两个频率最小的节点,合并它们,并将合并后的节点重新插入到优先队列中。
3. 重复上述步骤,直到队列中只剩下一个节点为止。该节点就是Huffman编码树的根节点。
4. 最后返回Huffman编码树的根节点。
这个函数返回一个指向HuffmanNode类型对象的指针,该指针指向Huffman编码树的根节点。
std::vector<std::vector<float>> weights 存入0.1 数据
std::vector<std::vector<float>> weights 是一个二维的向量,用来存储浮点数类型的数据。你可以使用以下代码将0.1存入weights中:
```cpp
#include <vector>
int main() {
std::vector<std::vector<float>> weights;
std::vector<float> innerVector;
innerVector.push_back(0.1);
weights.push_back(innerVector);
// 检查数据是否成功存入
for (const auto& inner : weights) {
for (const auto& value : inner) {
std::cout << value << " ";
}
std::cout << std::endl;
}
return 0;
}
```
这段代码首先创建了一个空的二维向量 weights,然后创建了一个内部的一维向量 innerVector,并将0.1存入其中。最后,将 innerVector 添加到 weights 中。通过遍历 weights,我们可以看到成功将0.1存入了 weights 中。
相关推荐
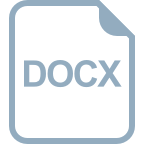













