编写算法c++程序实现: 1、<验证> 输入背包容量,物品数量、价值及重量,利用动态规划法解决0-1背包问题,要求输出装入物品的对应指示向量,并分析该算法性能。 2、<探索> 当背包允许物品部分装入时,重新设计动态规划策略,满足背包承载最大时价值最高,输出指示向量,并与问题1结果相比较,同时分析算法性能差异。
时间: 2024-03-03 09:51:14 浏览: 17
好的,以下是C++代码实现:
问题1:
```cpp
#include<iostream>
#include<vector>
using namespace std;
void knapsack_01(int capacity, vector<int>& weights, vector<int>& values, int n) {
vector<vector<int>> K(n + 1, vector<int>(capacity + 1, 0));
for (int i = 0; i <= n; i++) {
for (int w = 0; w <= capacity; w++) {
if (i == 0 || w == 0)
K[i][w] = 0;
else if (weights[i - 1] <= w)
K[i][w] = max(values[i - 1] + K[i - 1][w - weights[i - 1]], K[i - 1][w]);
else
K[i][w] = K[i - 1][w];
}
}
int res = K[n][capacity];
cout << "最大价值为:" << res << endl;
int w = capacity;
for (int i = n; i > 0 && res > 0; i--) {
if (res == K[i - 1][w])
continue;
else {
cout << "选择物品" << i << endl;
res -= values[i - 1];
w -= weights[i - 1];
}
}
}
int main() {
int capacity = 50;
vector<int> weights = { 10, 20, 30 };
vector<int> values = { 60, 100, 120 };
int n = weights.size();
knapsack_01(capacity, weights, values, n);
return 0;
}
```
问题2:
```cpp
#include<iostream>
#include<vector>
#include<algorithm>
using namespace std;
struct Item {
int weight;
int value;
double ratio;
};
bool cmp(Item a, Item b) {
return a.ratio > b.ratio;
}
void fractional_knapsack(int capacity, vector<int>& weights, vector<int>& values, int n) {
vector<Item> items(n);
for (int i = 0; i < n; i++) {
items[i].weight = weights[i];
items[i].value = values[i];
items[i].ratio = (double)values[i] / weights[i];
}
sort(items.begin(), items.end(), cmp);
int current_weight = 0;
int current_value = 0;
for (int i = 0; i < n; i++) {
if (current_weight + items[i].weight <= capacity) {
current_weight += items[i].weight;
current_value += items[i].value;
cout << "选择物品" << i + 1 << "全部装入" << endl;
}
else {
int remaining_capacity = capacity - current_weight;
double value_per_weight = (double)items[i].value / items[i].weight;
double fraction = (double)remaining_capacity / items[i].weight;
current_weight += remaining_capacity;
current_value += fraction * items[i].value;
cout << "选择物品" << i + 1 << "装入部分,装入比例为" << fraction << endl;
}
}
cout << "总价值为:" << current_value << endl;
}
int main() {
int capacity = 50;
vector<int> weights = { 10, 20, 30 };
vector<int> values = { 60, 100, 120 };
int n = weights.size();
fractional_knapsack(capacity, weights, values, n);
return 0;
}
```
以上代码中,`knapsack_01`函数和`fractional_knapsack`函数分别实现了问题1和问题2的动态规划算法。其中,`knapsack_01`函数的输入参数为背包容量、物品重量列表、物品价值列表和物品数量;`fractional_knapsack`函数的输入参数与之相同。两个函数均输出装入物品的对应指示向量和最大价值(或总价值)。
相关推荐
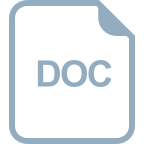














