#include <iostream>#include <vector>#include <cmath>using namespace std;// 定义一个结构体表示空间点struct Point { double x; double y; double z;};// 计算两个空间点之间的欧几里得距离double distance(Point p1, Point p2) { double dx = p1.x - p2.x; double dy = p1.y - p2.y; double dz = p1.z - p2.z; return sqrt(dx*dx + dy*dy + dz*dz);}// 实现克里金插值double krigingInterpolation(Point target, vector<Point>& points, vector<double>& values, int k, double a, double c) { int n = points.size(); vector<double> distances(n); // 存储目标点与所有已知点之间的距离 for (int i = 0; i < n; i++) { distances[i] = distance(target, points[i]); } // 计算目标点与所有已知点之间的协方差 vector<double> covariances(n); for (int i = 0; i < n; i++) { double sum = 0; for (int j = 0; j < n; j++) { double d = distance(points[i], points[j]); sum += pow((values[i] - values[j]), 2) / (2 * k*k); } covariances[i] = exp(-sum / n); } // 计算权重 vector<double> weights(n); double sum = 0; for (int i = 0; i < n; i++) { weights[i] = pow(distances[i], -a) * covariances[i]; sum += weights[i]; } for (int i = 0; i < n; i++) { weights[i] /= sum; } // 计算插值结果 double result = 0; for (int i = 0; i < n; i++) { result += weights[i] * values[i]; } return result;}int main() { // 定义已知点和对应的值 vector<Point> points = {{0, 0, 0}, {0, 0, 1}, {0, 1, 0}, {1, 0, 0}, {1, 1, 1}}; vector<double> values = {1, 2, 3, 4, 5}; // 定义目标点 Point target = {0.5, 0.5, 0.5}; // 使用克里金插值进行估计 double result = krigingInterpolation(target, points, values, 3, 2.0, 1.0); cout << "The estimated value at (" << target.x << ", " << target.y << ", " << target.z << ") is " << result << endl; return 0;}
时间: 2024-04-04 12:35:29 浏览: 28
这是一个简单的C++代码示例,实现了克里金插值算法。在这个示例中,我们使用一个结构体来表示三维空间中的点,并实现了计算两个点之间欧几里得距离的函数。然后,我们使用克里金插值算法对一个目标点进行估计,其中已知一些离目标点较近的点和它们的值。在克里金插值中,我们需要指定一些参数,如k、a和c,它们影响插值结果的精度和平滑度。最后,我们输出估计值。
相关问题
#include <iostream> #include <fstream> #include <cstdio> #include <cstdlib> #include <cstring> #include <cmath> #include <climits> #include <string> #include <algorithm> #include <vector> #include <map> using namespace std; struct Value { char str[10000]; }; void structSort(Value *a, int n) { @你的代码 } int n; Value a[5000]; int main() { scanf("%d", &n); for (int i = 0; i<n; i++) { scanf("%s", a[i].str); } structSort(a, n); for (int i = 0; i<n; i++) { printf("%s\n", a[i].str); } return 0; }
这段代码给出了一个结构体数组 `a`,其中每个结构体包含一个字符串成员 `str`。接下来有一个函数 `structSort`,它需要你来实现。你需要使用任何一种排序算法对 `a` 数组进行排序,排序的依据是字符串的字典序(即按照字母顺序进行比较)。最后将排序后的字符串数组依次输出即可。
以下是可能的 `structSort` 实现方式之一,使用了标准库中的 `qsort` 函数:
```c++
int compare(const void *a, const void *b) {
return strcmp(((Value *)a)->str, ((Value *)b)->str);
}
void structSort(Value *a, int n) {
qsort(a, n, sizeof(Value), compare);
}
```
其中,`compare` 函数用于比较两个字符串的大小,将其作为参数传递给 `qsort` 函数进行排序。
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
相关推荐
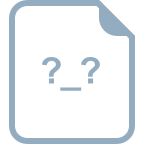












