state = torch.tensor(state[np.newaxis, :]).to(self.device)报错:tuple indices must be integers or slices, not tuple
时间: 2023-10-16 11:14:30 浏览: 153
这个错误通常是因为你使用了元组(tuple)来索引一个数组(array)或列表(list)。请确保你在使用索引时使用了整数或切片对象,而不是元组。
另外,如果你要将一个numpy数组转换为torch张量,请确保你的numpy数组是ndarray类型,而不是元组或其他类型。可能需要使用`np.asarray()`函数将元组转换为ndarray类型。
下面是一个使用整数索引的例子:
```
import torch
import numpy as np
state = np.array([1, 2, 3, 4])
state = torch.tensor(state[0]).to(torch.float32)
print(state)
```
输出:
```
tensor(1.)
```
如果你想使用切片对象索引,请使用类似于以下代码的方式:
```
import torch
import numpy as np
state = np.array([1, 2, 3, 4])
state = torch.tensor(state[:2]).to(torch.float32)
print(state)
```
输出:
```
tensor([1., 2.])
```
注意,在这个例子中,我们使用了切片`[:2]`来获取前两个元素。
相关问题
s = torch.tensor(s, device=self.device, dtype=torch.float32)
你的问题是关于使用PyTorch中的torch.tensor函数将数据转换为张量的代码。这个函数的作用是将给定的数据转换为张量,并可以指定设备和数据类型。在你的代码中,你将变量s转换为张量,并指定了设备为self.device,数据类型为torch.float32。
请注意,这只是根据你给出的代码片段进行的推测,如果你有更多的上下文信息或者具体的问题,我会尽力提供更详细的回答。
output_tensor = torch.tensor(net_output) ValueError: only one element tensors can be converted to Python scalars
这个错误通常出现在将多个元素的张量转换为 Python 标量时。如果你想要转换一个多维张量为 Python 标量,你需要指定一个特定的元素,如下所示:
```
output_tensor = torch.tensor(net_output)
scalar = output_tensor.item() # 获取第一个元素作为标量
```
如果你想要转换整个张量,可以使用 `numpy()` 函数将其转换为 NumPy 数组,然后再将其转换为 Python 列表:
```
output_tensor = torch.tensor(net_output)
output_list = output_tensor.numpy().tolist()
```
这将输出一个包含所有元素的 Python 列表。注意,这种方式只适用于张量中的所有元素都具有相同的类型和形状。如果你的张量具有不同的元素类型或形状,你需要使用其他方法来处理它们。
相关推荐
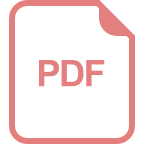
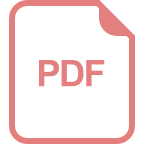
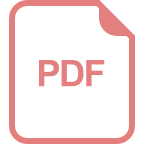













