帮我写一段取HTML网页内容并且存储到txt文档的代码
时间: 2023-08-31 21:42:25 浏览: 108
### 回答1:
答:我们可以使用Python来实现这个功能。下面是一段示例代码:import requests
from bs4 import BeautifulSoup# 这里填写你要获取内容的网页地址
url = 'http://example.com'# 使用requests库来获取网页内容
res = requests.get(url)# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(res.text, 'html.parser')# 获取网页内容
content = soup.get_text()# 将内容保存到txt文件中
with open('content.txt', 'w') as f:
f.write(content)
### 回答2:
以下是一个基于Python的示例代码,用于获取HTML网页内容并保存到txt文档:
```python
import requests
# 准备要爬取的网页链接
url = 'https://example.com/'
# 发送HTTP请求获取网页内容
response = requests.get(url)
html_content = response.text
# 将网页内容保存到txt文档
with open('web_content.txt', 'w', encoding='utf-8') as file:
file.write(html_content)
print('网页内容已保存到web_content.txt文件中。')
```
上述代码使用了Python的requests库来发送HTTP请求并获取网页内容。使用`get`函数获取网页内容时,会返回一个Response对象,我们可以通过`text`属性获取到网页的源码。
接下来,我们通过使用`open`函数打开一个名为web_content.txt的文件,在写模式下将网页内容写入到该文件中。这里要注意指定`encoding='utf-8'`来保证文本内容可以正确地写入和读取。
最后,通过`print`函数输出提示信息,表示网页内容已经成功保存到web_content.txt文件中。
你可以将上述代码保存为一个.py文件,并在运行该文件后,即可获取HTML网页内容并保存到txt文档中。
### 回答3:
下面是一个使用Python和BeautifulSoup库来获取HTML网页内容并存储到txt文档的代码:
```python
import requests
from bs4 import BeautifulSoup
def save_html_to_txt(url, file_path):
# 发送HTTP请求获取网页内容
response = requests.get(url)
html_content = response.text
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(html_content, 'html.parser')
# 获取网页正文内容
main_content = soup.get_text()
# 将正文内容写入txt文档
with open(file_path, 'w', encoding='utf-8') as file:
file.write(main_content)
# 设置目标网页的URL和要保存的txt文档路径
url = 'https://example.com'
file_path = 'output.txt'
# 调用函数将网页内容保存到txt文档
save_html_to_txt(url, file_path)
```
通过这段代码,你只需将`https://example.com`替换为你要获取内容的网页URL,并将`output.txt`替换为你想要保存的txt文档路径。执行代码后,网页内容将被提取并保存到指定的txt文档中。注意,你需要先安装`requests`和`BeautifulSoup`库才能运行上述代码。
相关推荐
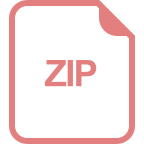
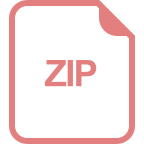
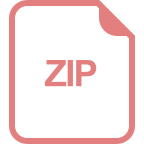
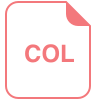
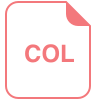
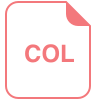
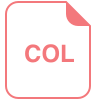
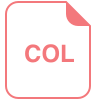









