用python生成一边录音一边显示FFT画布的多线程程序
时间: 2024-05-09 15:20:27 浏览: 10
以下是用Python生成一边录音一边显示FFT画布的多线程程序的示例代码:
```python
import pyaudio
import numpy as np
import matplotlib.pyplot as plt
import threading
# 创建PyAudio对象
p = pyaudio.PyAudio()
# 定义设置参数
CHUNK = 1024 # 每次读取的音频流的大小
FORMAT = pyaudio.paInt16 # 音频流的格式
CHANNELS = 1 # 单声道
RATE = 44100 # 采样率
# 初始化FFT画布
fig, ax = plt.subplots()
x = np.arange(0, CHUNK, 1)
line, = ax.plot(x, np.random.rand(CHUNK), '-', lw=2)
ax.set_xlim(0, CHUNK)
ax.set_ylim(-1, 1)
# 定义录音线程
class RecordThread(threading.Thread):
def __init__(self):
threading.Thread.__init__(self)
self.frames = []
def run(self):
stream = p.open(format=FORMAT, channels=CHANNELS, rate=RATE, input=True, frames_per_buffer=CHUNK)
while True:
data = stream.read(CHUNK)
self.frames.append(data)
def get_frames(self):
return self.frames
# 定义FFT线程
class FFTThread(threading.Thread):
def __init__(self, frames):
threading.Thread.__init__(self)
self.frames = frames
def run(self):
while True:
if len(self.frames) > 0:
data = self.frames.pop(0)
data = np.fromstring(data, dtype=np.int16)
y = np.fft.fft(data)
y = np.abs(y)
y = y / (CHUNK * 2 ** 16 / 2)
line.set_ydata(y)
plt.draw()
plt.pause(0.001)
# 启动录音线程和FFT线程
record_thread = RecordThread()
record_thread.start()
fft_thread = FFTThread(record_thread.get_frames())
fft_thread.start()
# 显示FFT画布
plt.show()
# 关闭PyAudio对象
p.terminate()
```
在这个程序中,我们使用了PyAudio库来读取音频流。我们使用两个线程来实现录音和FFT处理。录音线程不断读取音频流并将其存储到一个队列中。FFT线程不断从队列中获取音频流进行FFT处理,并更新画布。我们在画布上使用了matplotlib库来绘制FFT图形。最后,我们使用plt.show()方法来显示FFT画布,并关闭PyAudio对象。
相关推荐
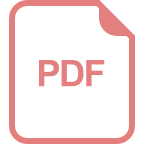
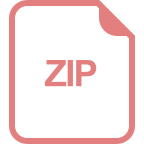
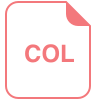








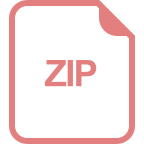
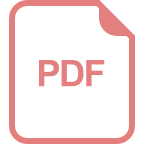
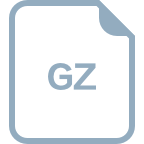
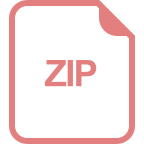
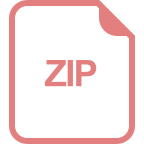