用python生成一边录音一边显示FFT动态画布的多线程程序
时间: 2024-05-03 19:21:57 浏览: 234
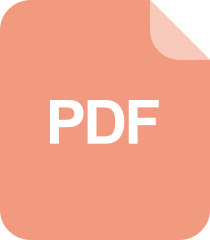
python实现录音小程序
以下是一份简单的Python多线程程序,用于录音并动态显示FFT:
```python
import pyaudio
import numpy as np
import matplotlib.pyplot as plt
import threading
class AudioRecorder(threading.Thread):
def __init__(self):
super(AudioRecorder, self).__init__()
self.chunk = 1024
self.rate = 44100
self.p = pyaudio.PyAudio()
self.stream = self.p.open(format=pyaudio.paFloat32, channels=1, rate=self.rate, input=True, frames_per_buffer=self.chunk)
self.running = True
def run(self):
while self.running:
data = np.fromstring(self.stream.read(self.chunk), dtype=np.float32)
self.fft(data)
def fft(self, data):
fig, ax = plt.subplots()
ax.set_xlim([0, self.rate / 2])
ax.set_ylim([-50, 50])
ax.set_xlabel('Frequency (Hz)')
ax.set_ylabel('Amplitude (dB)')
ax.set_title('Real-time FFT')
ax.grid(True)
fft_data = np.fft.fft(data)
fft_data = np.abs(fft_data[:int(len(fft_data) / 2)])
fft_data = 20 * np.log10(fft_data / np.max(fft_data))
frequencies = np.linspace(0, self.rate / 2, len(fft_data))
ax.plot(frequencies, fft_data)
plt.show(block=False)
plt.pause(0.001)
plt.clf()
def stop(self):
self.running = False
self.stream.stop_stream()
self.stream.close()
self.p.terminate()
if __name__ == '__main__':
recorder = AudioRecorder()
recorder.start()
input('Press enter to stop recording...')
recorder.stop()
```
此程序使用PyAudio库录制音频,并在FFT动态画布中显示每个块的FFT。每个块的大小可以通过调整“chunk”变量来更改。在程序运行时,可以通过按Enter键来停止录音并关闭程序。
注意:此程序仅用于参考,并可能需要进行修改才能满足您的需求。
阅读全文
相关推荐
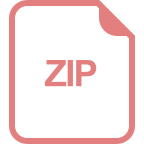








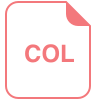
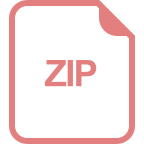
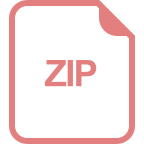
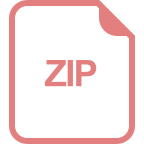
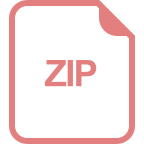