java弹窗表格实现管理员对图书的查询和修改,不用数据库
时间: 2024-03-14 07:48:28 浏览: 53
可以使用Java的Swing组件来实现管理员对图书的查询和修改,并且不用数据库。具体来说,可以使用JTable组件来实现表格的显示和编辑,使用JOptionPane组件来实现弹窗的显示。以下是简单的示例代码:
```
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.util.ArrayList;
public class BookManager {
private ArrayList<Book> books;
public BookManager() {
books = new ArrayList<Book>();
}
public void addBook(Book book) {
books.add(book);
}
public ArrayList<Book> searchBook(String keyword) {
ArrayList<Book> results = new ArrayList<Book>();
for (Book book : books) {
if (book.getTitle().contains(keyword) || book.getAuthor().contains(keyword)) {
results.add(book);
}
}
return results;
}
public void modifyBook(Book book) {
int index = books.indexOf(book);
if (index != -1) {
books.set(index, book);
}
}
public static void main(String[] args) {
BookManager manager = new BookManager();
JFrame frame = new JFrame("图书管理系统");
frame.setSize(800, 600);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new BorderLayout());
JTable table = new JTable(new DefaultTableModel(new Object[] {"书名", "作者", "出版社", "价格"}, 0));
JScrollPane scrollPane = new JScrollPane(table);
panel.add(scrollPane, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
JButton addButton = new JButton("添加图书");
addButton.addActionListener(e -> {
String title = JOptionPane.showInputDialog(frame, "请输入书名:");
String author = JOptionPane.showInputDialog(frame, "请输入作者:");
String publisher = JOptionPane.showInputDialog(frame, "请输入出版社:");
double price = Double.parseDouble(JOptionPane.showInputDialog(frame, "请输入价格:"));
Book book = new Book(title, author, publisher, price);
manager.addBook(book);
DefaultTableModel model = (DefaultTableModel) table.getModel();
model.addRow(new Object[] {book.getTitle(), book.getAuthor(), book.getPublisher(), book.getPrice()});
});
buttonPanel.add(addButton);
JButton searchButton = new JButton("查询图书");
searchButton.addActionListener(e -> {
String keyword = JOptionPane.showInputDialog(frame, "请输入关键字:");
ArrayList<Book> results = manager.searchBook(keyword);
if (results.size() == 0) {
JOptionPane.showMessageDialog(frame, "未找到相关图书!");
} else {
DefaultTableModel model = (DefaultTableModel) table.getModel();
model.setRowCount(0);
for (Book book : results) {
model.addRow(new Object[] {book.getTitle(), book.getAuthor(), book.getPublisher(), book.getPrice()});
}
}
});
buttonPanel.add(searchButton);
JButton modifyButton = new JButton("修改图书");
modifyButton.addActionListener(e -> {
int row = table.getSelectedRow();
if (row == -1) {
JOptionPane.showMessageDialog(frame, "请先选择要修改的图书!");
return;
}
String title = JOptionPane.showInputDialog(frame, "请输入书名:", table.getValueAt(row, 0));
String author = JOptionPane.showInputDialog(frame, "请输入作者:", table.getValueAt(row, 1));
String publisher = JOptionPane.showInputDialog(frame, "请输入出版社:", table.getValueAt(row, 2));
double price = Double.parseDouble(JOptionPane.showInputDialog(frame, "请输入价格:", table.getValueAt(row, 3)));
Book book = new Book(title, author, publisher, price);
manager.modifyBook(book);
DefaultTableModel model = (DefaultTableModel) table.getModel();
model.setValueAt(book.getTitle(), row, 0);
model.setValueAt(book.getAuthor(), row, 1);
model.setValueAt(book.getPublisher(), row, 2);
model.setValueAt(book.getPrice(), row, 3);
});
buttonPanel.add(modifyButton);
panel.add(buttonPanel, BorderLayout.SOUTH);
frame.setContentPane(panel);
frame.setVisible(true);
}
}
class Book {
private String title;
private String author;
private String publisher;
private double price;
public Book(String title, String author, String publisher, double price) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.price = price;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String toString() {
return "书名:" + title + ",作者:" + author + ",出版社:" + publisher + ",价格:" + price;
}
}
```
这个示例代码实现了一个简单的图书管理系统,其中BookManager类负责管理图书信息,包括添加、查询和修改,Book类代表图书实体,包括书名、作者、出版社和价格等属性。在程序运行时,通过Swing组件构建了一个包含表格和按钮的窗口,用户可以通过按钮进行相应的操作。由于图书信息存储在内存中的ArrayList中,所以不需要使用数据库。
阅读全文
相关推荐
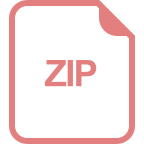








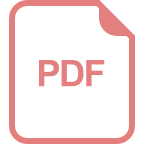
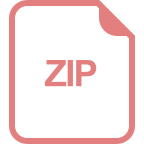
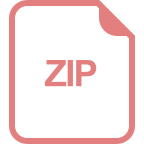
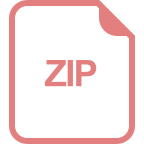
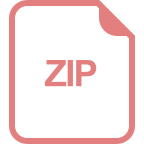
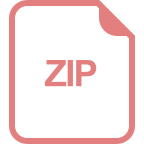
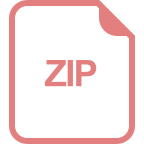
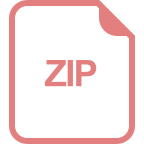