shell脚本 判断包含字符
时间: 2024-11-25 09:18:28 浏览: 25
在Shell脚本中,如果你想判断字符串是否包含某个字符或者子串,可以使用内置的字符串操作函数或者条件语句。这里有几个常见的方法:
1. **使用`[[ ... ]]`条件表达式**:
```bash
string="Hello, World!"
if [[ "$string" =~ [c] ]]; then
echo "包含字符 'c'"
else
echo "不包含字符 'c'"
fi
```
这里使用正则表达式`=~`来匹配字符或子串。
2. **使用`echo`和`grep`**:
```bash
string="Hello, World!"
if echo "$string" | grep -q "[c]"; then
echo "包含字符 'c'"
else
echo "不包含字符 'c'"
fi
```
`grep -q`用于安静模式查找,如果找到就会返回真。
3. **使用`test`或`[ ]`数组测试**:
```bash
string="Hello, World!"
if test "${string}" = *"[c]*"; then
echo "包含字符 'c'"
else
echo "不包含字符 'c'"
fi
```
这里利用了shell的globbing特性,`*`代表零个或多个任意字符。
相关问题
shell脚本判断分割字符串
在Shell脚本中,可以使用各种方法来判断和分割字符串。以下是一些常用的方法:
1. 使用if语句进行字符串比较:
```shell
if [ "$str1" = "$str2" ]; then
echo "字符串相等"
else
echo "字符串不相等"
fi
```
2. 使用正则表达式进行字符串匹配:
```shell
if [[ "$str" =~ pattern ]]; then
echo "字符串匹配"
else
echo "字符串不匹配"
fi
```
3. 使用通配符进行字符串模式匹配:
```shell
if [[ "$str" == *pattern* ]]; then
echo "字符串匹配"
else
echo "字符串不匹配"
fi
```
4. 使用内置命令expr进行字符串分割:
```shell
str="hello,world"
delimiter=","
result=$(expr "$str" : '\(.*'$delimiter'\)')
echo "$result" # 输出"hello,"
```
5. 使用内置命令cut进行字符串分割:
```shell
str="hello,world"
delimiter=","
result=$(echo "$str" | cut -d "$delimiter" -f 1)
echo "$result" # 输出"hello"
```
6. 使用内置命令IFS进行字符串分割:
```shell
str="hello,world"
IFS=',' read -ra parts <<< "$str"
for part in "${parts[@]}"; do
echo "$part"
done
# 输出:
# hello
# world
```
以上是一些常用的Shell脚本判断和分割字符串的方法。根据具体的需求,可以选择适合的方法来处理字符串操作。
shell脚本判断某个字符串是否包含特定字符串
以下是Shell脚本判断某个字符串是否包含特定字符串的方法:
```shell
strA="hello world"
strB="world"
result=$(echo $strA | grep $strB)
if [[ "$result" != "" ]]; then
echo "strA包含strB"
else
echo "strA不包含strB"
fi
```
上述代码中,首先定义了两个字符串变量`strA`和`strB`,然后使用`grep`命令在`strA`中查找`strB`,将结果保存在变量`result`中。如果`result`不为空,则说明`strA`包含`strB`,否则不包含。
阅读全文
相关推荐
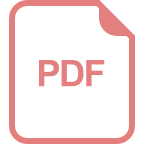
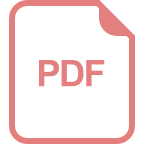
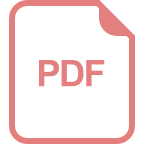













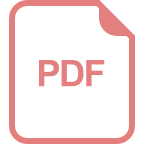