do not have corresponding entries in the value map
时间: 2024-04-25 21:22:23 浏览: 30
回答: 如果在值映射中没有对应的条目,那么根据引用\[1\]和引用\[3\]的描述,返回的值将是null。这意味着在映射中没有为该键提供任何映射。根据引用\[2\]的描述,映射不能包含重复的键,每个键最多只能映射到一个值。因此,如果在值映射中没有对应的条目,那么返回的值将是null。
#### 引用[.reference_title]
- *1* *2* *3* [【跟着源码学英语】 Map.java 是个接口](https://blog.csdn.net/Aran_/article/details/113941084)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关问题
The source ip address does not have a corresponding interface
### 回答1:
这个错误通常出现在网络配置上,它是指某个设备或主机正在尝试使用一个没有对应网络接口的IP地址。这可能是由于网络接口未正确配置,或者网络拓扑发生了变化,导致IP地址无法正常映射到网络接口。要解决这个问题,可以检查网络配置,确保IP地址与网络接口匹配,并确保网络拓扑没有发生变化。如果问题仍然存在,可能需要进行更深入的网络故障排除。
### 回答2:
源IP地址没有相应的接口,是指在网络通信过程中发现源IP地址与其应该发送的接口不匹配的情况。
在计算机网络中,每个网络设备都有一个或多个网络接口,用于与其他设备进行通信。每个接口都有一个对应的IP地址,用于标识该设备在网络中的位置。当设备收到一个数据包时,会根据目标IP地址来确定数据包的传输路径,并将其发送到相应的接口。
然而,有时候会出现源IP地址与其应该发送的接口不匹配的情况。这可能是因为配置错误、路由表错误或者网络故障等原因导致的。当发生这种情况时,设备无法将数据包正确地发送到目标设备,从而导致通信失败。
要解决这个问题,首先需要检查设备的网络配置和路由表是否正确。可以通过查看设备的IP地址和子网掩码、检查路由表是否包含正确的目标网络等方式来排查问题。如果网络配置和路由表正确,那么可能是网络中的其他设备或网络设备出现故障,需要对网络进行深入诊断和排查。
总之,源IP地址没有相应的接口是网络通信中的一种错误情况,需要通过检查配置和路由表,或者对网络进行故障排查来解决。
### 回答3:
这句话是在网络中出现的错误信息,意思是来源IP地址没有相应的接口。
在计算机网络中,每个设备都有一个或多个网络接口,用于与其他设备通信。为了使网络通信流畅,每个网络接口都会被分配一个或多个IP地址。当一个设备收到来自另一个设备的数据包时,它会根据数据包中的源IP地址找到相应的接口,然后将数据包发送到该接口。
然而,有时候会出现"source ip address does not have a corresponding interface"的错误。这可能是由以下情况引起的:
1. IP地址配置错误:源IP地址可能是错误的,或者没有正确地配置。在这种情况下,设备无法找到与该地址对应的接口,因此会报错。
2. 网络配置错误:可能存在网络配置问题,例如路由表配置错误或网络拓扑变化导致设备无法找到与源IP地址相对应的接口。
要解决这个问题,可以尝试以下步骤:
1. 检查IP地址配置:确保源IP地址是正确的,并且已经正确配置在设备上。
2. 检查网络配置:检查设备的路由表和网络拓扑,并确保没有任何配置错误或变化导致设备无法找到接口。
3. 重启设备:有时候,重新启动设备可以解决临时的网络配置问题。
如果以上步骤都无法解决问题,可能需要进一步检查网络设备和配置,或者联系网络管理员以获取帮助。
Not enough information to list load addresses in the image map.
This error message typically occurs when trying to load an executable file that doesn't contain enough information about its load addresses. The image map is a table that maps the virtual addresses in the executable file to the corresponding physical addresses when the program is loaded into memory. If this information is missing or incomplete, the loader cannot determine where to load the program into memory, and the error message is displayed.
There are several possible causes for this error message, such as an incorrect format of the executable file, a corrupted file, or a mismatch between the file and the operating system or architecture. To resolve this issue, you may need to check the file format and version, verify the integrity of the file, or consult the documentation or support resources for the software or operating system you are using.
相关推荐
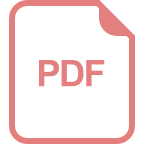
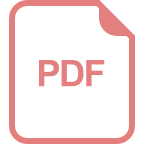
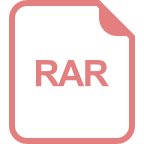













