如何使用C++指针编写一个程序来实现以下功能:首先,提示用户输入学生的名字和考试分数;然后,计算并输出整个班级的平均分;接着,找出并显示所有考试分数低于平均分的学生的姓名和对应分数;最后,找到并输出最高的考试分数以及对应的学员姓名。这个程序应该如何设计和组织?
时间: 2024-12-22 14:19:17 浏览: 11
为了实现这个功能,我们可以创建一个名为`Student`的结构体或类,包含学生的姓名和分数。然后,我们可以定义一个动态数组(vector)来存储整个班级的学生信息,因为我们需要添加、删除和查找元素。下面是程序的主要步骤:
1. 定义`Student`类/结构体:
```cpp
#include <iostream>
#include <string>
#include <vector>
class Student {
public:
std::string name;
double score;
// 构造函数和获取名字和分数的方法
Student(const std::string& n, double s) : name(n), score(s) {}
std::string getName() const { return name; }
double getScore() const { return score; }
};
```
2. 创建一个动态数组来存储学生:
```cpp
std::vector<Student> students;
```
3. 提示用户输入学生数据并添加到数组中:
```cpp
int main() {
int numStudents;
std::cout << "Enter the number of students: ";
std::cin >> numStudents;
for (int i = 0; i < numStudents; ++i) {
std::string name;
double score;
std::cout << "Enter student " << i+1 << "'s name and score: ";
std::cin >> name >> score;
students.push_back(Student(name, score));
}
// ...
}
```
4. 计算平均分并输出:
```cpp
double averageScore = 0.0;
for (const auto& student : students) {
averageScore += student.getScore();
}
averageScore /= numStudents;
std::cout << "The class average score is: " << averageScore << "\n";
```
5. 找出并显示分数低于平均分的学生:
```cpp
std::vector<Student> belowAverage;
for (const auto& student : students) {
if (student.getScore() < averageScore) {
belowAverage.push_back(student);
}
}
if (!belowAverage.empty()) {
std::cout << "Students with scores below average:\n";
for (const auto& student : belowAverage) {
std::cout << student.getName() << ": " << student.getScore() << "\n";
}
} else {
std::cout << "No students have a score below the average.\n";
}
```
6. 找到并输出最高分数及其对应的学生姓名:
```cpp
if (students.size() > 0) {
auto maxScoreIndex = std::max_element(students.begin(), students.end(),
[](const auto& a, const auto& b) -> bool {
return a.getScore() < b.getScore();
});
Student* maxScoreStudent = maxScoreIndex == students.end() ? nullptr : &(*maxScoreIndex);
if (maxScoreStudent) {
std::cout << "The highest score is by " << maxScoreStudent->getName()
<< " with a score of " << maxScoreStudent->getScore() << ".\n";
} else {
std::cout << "There are no students to compare for the highest score.\n";
}
} else {
std::cout << "The list is empty.\n";
}
```
阅读全文
相关推荐
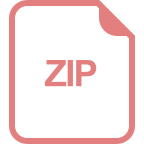
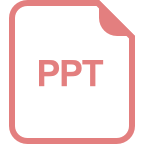
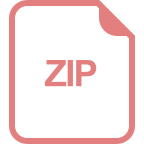
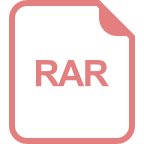
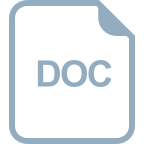
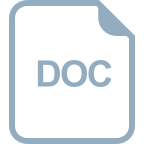












