随机森林的n_estimators和max_features什么意思
时间: 2023-11-21 07:05:21 浏览: 68
随机森林是一种集成学习算法,它由多个决策树组成。其中,n_estimators表示随机森林中包含的决策树数量,max_features表示每个决策树在训练时,从所有特征中选择的最大特征数。在实际应用中,选择合适的n_estimators和max_features可以对随机森林的性能产生影响。一般来说,随机森林中的n_estimators越多,模型的准确性越高,但训练时间也会增加。而max_features的选择可以控制决策树的多样性,较小的max_features可以让每个决策树更加不同,从而提高模型的泛化性能。
相关问题
#这里给模型需要的max_depth/n_estimators/max_features/min_samples_split的参数范围
好的,下面是一些常用的决策树和随机森林的超参数范围:
决策树:
- max_depth:树的最大深度,一般设为10-20,范围可以是1到无穷大。
- min_samples_split:分裂内部节点所需的最小样本数,一般设为2-10,范围可以是1到任意大的整数。
- min_samples_leaf:每个叶节点所需的最小样本数,一般设为1-5,范围可以是1到任意大的整数。
随机森林:
- n_estimators:森林中树的数量,一般设为100-500,范围可以是1到任意大的整数。
- max_depth:每棵树的最大深度,一般设为10-20,范围可以是1到无穷大。
- max_features:每个节点在分裂时使用的特征数,一般设为sqrt(n_features),其中n_features是总特征数,也可以使用log2(n_features)。范围可以是1到n_features。
- min_samples_split:分裂内部节点所需的最小样本数,一般设为2-10,范围可以是1到任意大的整数。
- min_samples_leaf:每个叶节点所需的最小样本数,一般设为1-5,范围可以是1到任意大的整数。
需要注意的是,超参数的合理范围也取决于数据集的大小和复杂度。上述范围仅供参考,具体范围需要根据实际情况进行调整。
随机森林 n estimators
随机森林中的参数n_estimators表示构建的决策树的数量。每个决策树都是通过对原始数据集进行自助采样得到的,因此每个决策树都是基于不同的数据集构建的。通过增加n_estimators的值,可以增加随机森林的复杂度和预测能力,但也会增加计算成本。一般来说,增加n_estimators可以提高模型的稳定性和准确性,但在一定程度上也会增加过拟合的风险。\[1\]\[3\]
#### 引用[.reference_title]
- *1* [SKlearn学习笔记——随机森林](https://blog.csdn.net/luanfenlian0992/article/details/106476238)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [随机森林 n_estimators参数 max_features参数](https://blog.csdn.net/u012768474/article/details/92829985)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
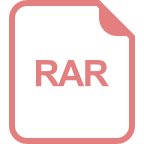












