定义抽象类 Shape 及其子类 Circle 和 Rectangle, 要求: (1) 抽象类 Shape 具有纯虚函数 Area(), 用于计算其子类的面积; (2) 抽象类 Shape 的子类 Circle、Rectangle, 覆盖父类的 Area() 方法, 用于计算各自的面积; (3) 通过 Shape 类指针分别指向 Circle 对象、Rectagnle 对象, 并调用 Aera() 方法用于输出面积. 示例: #include <string> #include <iostream> #define _USE_MATH_DEFINES #include <math.h> class Shape { public: virtual double Area() = 0; }; class Circle: public Shape { public: Circle(double r); virtual double Area(); protected: double r; }; Circle::Circle(double r) { ... } double Circle::Area() { ... } 1 class Rectangle: public Shape { public: Rectangle(double w, double h); virtual double Area(); protected: double w; double h; }; Rectangle::Rectangle(double w, double h) { ... } double Rectangle::Area() { ... } int main() { std::cout << "-------------" << std::endl; std::cout << "创建Circel对象:" << std::endl; Circle circle(1); std::cout << " 面 积 为 :" << circle.Area() << std::endl; std::cout << "-------------" << std::endl; std::cout << "创建Rectangle对象:" << std::endl; Rectangle rect(2,2); std::cout << " 面 积 为 :" << rect.Area() << std::endl; Shape * pt; std::cout << "-------------" << std::endl; std::cout << "Shape 指 针 引 用 Circle 对 象 :" << std::endl; pt = &circle; std::cout << " 面 积 为 :" << pt->Area() << std::endl; std::cout << "-------------" << std::endl; std::cout << "Shape 指 针 引 用 Rectangle 对 象 :" << std::endl; pt = ▭ std::cout << " 面 积 为 :" << pt->Area() << std::endl; return 0; }
时间: 2024-04-23 16:22:22 浏览: 218
好的,这是一个简单的面向对象编程题目,看起来你已经掌握了抽象类和继承的概念。
代码中的抽象类 Shape 拥有一个纯虚函数 Area(),用于计算其子类的面积。子类 Circle 和 Rectangle 分别继承了 Shape 并覆盖了 Area() 方法,用于计算各自的面积。在 main 函数中,分别创建了 Circle 和 Rectangle 对象,并使用 Shape 类型的指针指向这些对象并调用 Area() 方法输出面积。
这是一个良好的面向对象设计,使用抽象类确保了子类必须实现 Area() 方法,保证了代码的可维护性和可扩展性。
如果你对代码中的细节还有疑问,可以继续深入学习面向对象编程的相关知识。
相关问题
定义抽象类 Shape 及其子类 Circle 和 Rectangle, 要求: (1) 抽象类 Shape 具有纯虚函数 Area(), 用于计算其子类的面积; (2) 抽象类 Shape 的子类 Circle、Rectangle, 覆盖父类的 Area() 方法, 用于计算各自的面积; (3) 通过 Shape 类指针分别指向 Circle 对象、Rectagnle 对象, 并调用 Aera() 方法用于输出面积.
好的,以下是实现 Shape 抽象类及其子类 Circle 和 Rectangle 的代码:
```cpp
#include<iostream>
using namespace std;
// Shape 抽象类,定义了纯虚函数 Area(),用于计算其子类的面积
class Shape {
public:
virtual double Area() = 0; // 纯虚函数
};
// Circle 类,继承自 Shape 类
class Circle : public Shape {
public:
Circle(double r) : m_Radius(r) {} // 构造函数
virtual double Area() { return 3.14 * m_Radius * m_Radius; } // 重写 Area() 方法,计算圆的面积
private:
double m_Radius; // 圆的半径
};
// Rectangle 类,继承自 Shape 类
class Rectangle : public Shape {
public:
Rectangle(double l, double w) : m_Length(l), m_Width(w) {} // 构造函数
virtual double Area() { return m_Length * m_Width; } // 重写 Area() 方法,计算矩形的面积
private:
double m_Length; // 矩形的长度
double m_Width; // 矩形的宽度
};
int main()
{
Shape* pShape = NULL;
// Circle 对象
Circle circle(5);
pShape = &circle;
cout << "Circle's area: " << pShape->Area() << endl;
// Rectangle 对象
Rectangle rectangle(4, 6);
pShape = &rectangle;
cout << "Rectangle's area: " << pShape->Area() << endl;
return 0;
}
```
输出结果为:
```
Circle's area: 78.5
Rectangle's area: 24
```
在程序中,我们首先定义了一个抽象类 Shape,其中只有一个纯虚函数 Area(),表示计算面积的方法。然后我们分别定义了 Circle 和 Rectangle 两个子类,继承自 Shape 类,并重写 Area() 方法,分别计算圆和矩形的面积。在 main() 函数中,我们定义一个 Shape 类型的指针 pShape,然后通过它分别指向 Circle 和 Rectangle 对象,并调用 Area() 方法输出面积。
定义抽象类 Shape 及其子类Circle 和 Rectangle,要求: (1)抽象类Shape 具有纯虛函数 Area(),用于计算其子类的面积; (2)抽象类Shape的子类 Circle、Rectangle,覆盖父类的Area()方法,用于计算各自的面积; (3)通过Shape 类指针分別指向 Circle对象、Rectagnle 对象,并调用Aera()方法用于输出面积。
下面是实现上述要求的示例代码:
```cpp
#include <iostream>
using namespace std;
// 抽象类 Shape
class Shape {
public:
// 纯虚函数,用于计算面积
virtual double Area() = 0;
};
// Circle 类,继承自 Shape
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
// 重写父类 Area() 方法,计算圆的面积
double Area() {
return 3.14159 * radius * radius;
}
private:
double radius;
};
// Rectangle 类,继承自 Shape
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
// 重写父类 Area() 方法,计算矩形的面积
double Area() {
return width * height;
}
private:
double width;
double height;
};
int main() {
// 通过 Shape 类指针分别指向 Circle 对象、Rectangle 对象
Shape *shape1 = new Circle(2.0);
Shape *shape2 = new Rectangle(3.0, 4.0);
// 调用各自的 Area() 方法计算面积并输出
cout << "Circle area: " << shape1->Area() << endl;
cout << "Rectangle area: " << shape2->Area() << endl;
delete shape1;
delete shape2;
return 0;
}
```
在上述代码中,Shape类是一个抽象类,其中定义了纯虚函数Area(),用于计算其子类的面积。Circle类和Rectangle类是Shape类的子类,分别覆盖了父类的Area()方法,用于计算各自的面积。在main()函数中,通过Shape类指针指向Circle对象和Rectangle对象,并分别调用了各自的Area()方法,用于计算和输出面积。
阅读全文
相关推荐
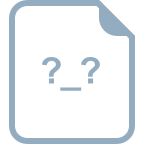
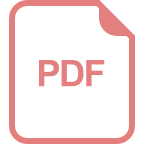
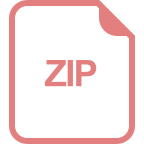













