假设我要flume采集数据到mysql,假设要采集的数据格式为文本格式,并且自定义一个mysqlsink,该怎么实现,帮我写个java文件和pom.xml依赖文件
时间: 2024-05-07 16:22:37 浏览: 109
以下是一个简单的Flume配置,它使用自定义的MySQL sink 来将文本数据写入MySQL。
首先,创建一个Java文件,命名为MySQLSink.java,包含以下内容:
```java
package com.example.flume;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import org.apache.flume.Context;
import org.apache.flume.Event;
import org.apache.flume.conf.Configurable;
import org.apache.flume.sink.AbstractSink;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MySQLSink extends AbstractSink implements Configurable{
private static final Logger logger = LoggerFactory.getLogger(MySQLSink.class);
private String driver;
private String url;
private String username;
private String password;
private String tableName;
private PreparedStatement preparedStatement = null;
private Connection connection = null;
public void configure(Context context) {
driver = context.getString("driver");
url = context.getString("url");
username = context.getString("username");
password = context.getString("password");
tableName = context.getString("tableName");
}
public synchronized void start() {
try {
Class.forName(driver);
connection = DriverManager.getConnection(url, username, password);
String sql = "INSERT INTO " + tableName + "(data) VALUES(?)";
preparedStatement = connection.prepareStatement(sql);
super.start();
} catch (ClassNotFoundException e) {
logger.error("Can not find JDBC Driver!", e);
throw new RuntimeException(e);
} catch (SQLException e) {
logger.error("Can not create Connection!", e);
throw new RuntimeException(e);
}
}
public synchronized void stop() {
try {
preparedStatement.close();
connection.close();
} catch (SQLException e) {
logger.error("Error while closing the connection!", e);
}
super.stop();
}
public Status process() {
Status status = null;
List<String> dataList = new ArrayList<String>();
Channel channel = getChannel();
Transaction transaction = channel.getTransaction();
transaction.begin();
try {
Event event = channel.take();
while (event != null) {
String data = new String(event.getBody());
dataList.add(data);
event = channel.take();
}
if (dataList.size() != 0) {
for (String data : dataList) {
preparedStatement.setString(1, data);
preparedStatement.addBatch();
}
preparedStatement.executeBatch();
connection.commit();
}
transaction.commit();
status = Status.READY;
} catch (Exception e) {
transaction.rollback();
logger.error("Failed to log event!", e);
status = Status.BACKOFF;
} finally {
transaction.close();
}
return status;
}
}
```
然后,创建一个pom.xml依赖文件,包含以下内容:
```xml
<dependencies>
<dependency>
<groupId>org.apache.flume</groupId>
<artifactId>flume-ng-core</artifactId>
<version>1.6.0</version>
</dependency>
<dependency>
<groupId>org.apache.flume</groupId>
<artifactId>flume-ng-sdk</artifactId>
<version>1.6.0</version>
</dependency>
<dependency>
<groupId>org.apache.flume</groupId>
<artifactId>flume-ng-configuration</artifactId>
<version>1.6.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
</dependencies>
```
在Flume配置文件中添加以下内容:
```properties
# Flume source, channel, and sink configurations
agent.sources = source1
agent.channels = channel1
agent.sinks = sink1
# Source configuration
agent.sources.source1.type = exec
agent.sources.source1.command = tail -f /var/log/syslog
# Channel configuration
agent.channels.channel1.type = memory
agent.channels.channel1.capacity = 1000
agent.channels.channel1.transactionCapacity = 100
# Sink configuration
agent.sinks.sink1.type = com.example.flume.MySQLSink
agent.sinks.sink1.driver = com.mysql.jdbc.Driver
agent.sinks.sink1.url = jdbc:mysql://localhost:3306/test
agent.sinks.sink1.username = root
agent.sinks.sink1.password = password
agent.sinks.sink1.tableName = logs
agent.sinks.sink1.channel = channel1
# Bind the source and sink to the channel
agent.sources.source1.channels = channel1
agent.sinks.sink1.channel = channel1
```
在这个配置中,我们使用exec源来读取一个文本文件的最新内容。然后,我们将其发送到内存通道中,并使用自定义的MySQL sink 将其写入MySQL表中。请注意,MySQLSink中的配置参数会从Flume配置文件中读取。在此示例中,我们将数据写入名为logs的表中。
注意:请将示例中的数据库连接信息更改为适合您环境的值。
阅读全文
相关推荐
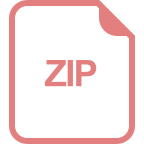
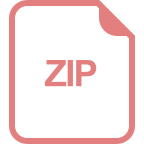
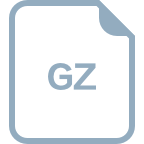

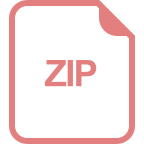
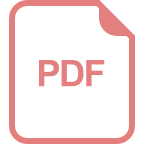
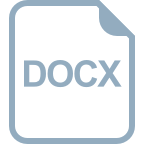
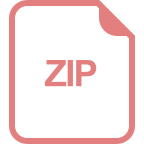
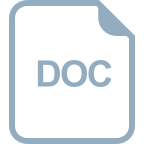









