C# 调用C语言dll返回结构体
时间: 2023-06-16 10:06:09 浏览: 100
在C#中调用C语言编写的DLL,可以使用DllImport特性来导入DLL中的函数。如果需要返回结构体,可以使用Marshal.PtrToStructure方法将指针转换为结构体。
以下是一个示例代码:
```c
// C语言编写的DLL代码
struct MyStruct {
int a;
float b;
char c[10];
};
__declspec(dllexport) void getStruct(struct MyStruct* ptr) {
ptr->a = 1;
ptr->b = 2.0f;
strcpy_s(ptr->c, "hello");
}
```
```csharp
// C#代码
using System;
using System.Runtime.InteropServices;
[StructLayout(LayoutKind.Sequential)]
public struct MyStruct {
public int a;
public float b;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 10)]
public string c;
};
class Program {
[DllImport("mydll.dll")]
private static extern void getStruct(ref MyStruct s);
static void Main(string[] args) {
MyStruct s = new MyStruct();
getStruct(ref s);
Console.WriteLine(s.a);
Console.WriteLine(s.b);
Console.WriteLine(s.c);
}
}
```
需要注意的是,结构体在C#中的定义需要使用StructLayout特性,并指定LayoutKind.Sequential。同时,结构体中的字符数组需要使用MarshalAs特性,并指定字符数组的长度和类型。在调用C语言DLL导出函数时,需要使用ref关键字将结构体传递给函数。
相关推荐
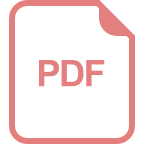
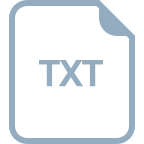











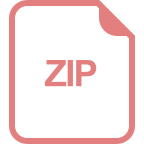