用Python爬虫对全国大学前十名数据进行爬取,并可视化展示
时间: 2024-01-18 07:03:07 浏览: 121
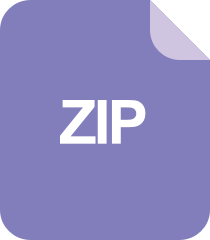
基于python的网络爬虫爬取天气数据及可视化分析python大作业.zip

好的,下面是具体步骤:
1. 分析目标网站
首先我们需要确定要爬取的网站,这里我们以“中国最好大学排名”为例,使用 https://www.shanghairanking.cn/rankings/bcur/2021 这个网站来获取前十名大学的相关数据。
2. 确定爬取方式
我们可以使用 Python 的 requests 库来发送 HTTP 请求,获取网页源代码,然后使用 BeautifulSoup 库来解析 HTML 文档,从而获取我们需要的数据。
3. 编写爬虫程序
下面是基于 requests 和 BeautifulSoup 的爬虫程序示例,可以获取前十名大学的排名、名称、所在地和排名得分。
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.shanghairanking.cn/rankings/bcur/2021'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
table = soup.find('table', class_='rk-table')
rows = table.find_all('tr')
data = []
for row in rows[1:11]:
cols = row.find_all('td')
rank = cols[0].text.strip()
name = cols[1].text.strip()
location = cols[2].text.strip()
score = cols[3].text.strip()
data.append((rank, name, location, score))
```
4. 数据可视化展示
我们可以使用 Python 的 matplotlib 库来进行数据可视化展示,下面是基于 matplotlib 的代码示例,可以将获取到的前十名大学数据进行可视化展示。
```python
import matplotlib.pyplot as plt
names = [d[1] for d in data]
scores = [float(d[3]) for d in data]
plt.bar(names, scores)
plt.title('Top Ten Universities in China')
plt.xlabel('University')
plt.ylabel('Score')
plt.show()
```
运行程序后,我们可以得到一个简单的柱状图,展示了前十名大学的排名得分。

完整代码如下:
阅读全文
相关推荐
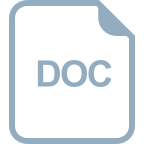
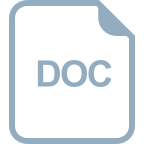

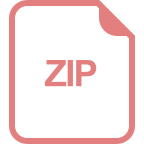
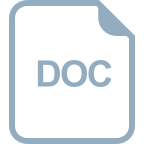
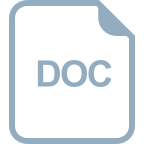
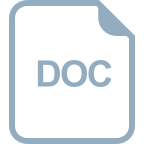



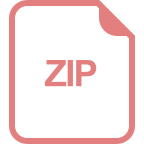



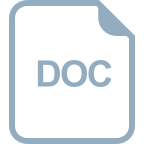
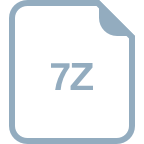
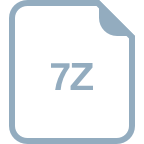