localStorage.setItem('token', {'username':name})
时间: 2023-10-02 20:13:23 浏览: 95
This line of code will store a JavaScript object as a value for the 'token' key in the browser's local storage. The object has a property 'username' whose value is the variable 'name'.
However, the value passed to the setItem method should be a string, so the object needs to be converted to a string using a method such as JSON.stringify() before being stored.
Corrected code:
```
localStorage.setItem('token', JSON.stringify({'username':name}));
```
相关问题
import axios from "axios"; export default { name:"UserLogin", data() { return { loginForm: { username: "", password: "", }, registerDialogVisible: false, registerForm: { username: "", password: "", }, }; }, methods: { showRegisterDialog() { this.registerDialogVisible = true; }, async submitRegisterForm(formName) { this.$refs[formName].validate(async (valid) => { if (valid) { try { await axios.post("http://localhost:5001/register", { username: this.registerForm.username, password: this.registerForm.password, }); // 自动登录 this.loginForm.username = this.registerForm.username; this.loginForm.password = this.registerForm.password; await this.submitForm("loginForm"); this.registerDialogVisible = false; } catch (error) { console.log(error); this.$message({ message: "Registration failed!", type: "error", }); } } else { this.$message({ message: "Invalid input!", type: "error", }); return false; } }); }, async submitForm(formName) { this.$refs[formName].validate(async (valid) => { if (valid) { try { const response = await axios.post("http://localhost:5001/login", { username: this.loginForm.username, password: this.loginForm.password, }); const token = response.data.token; const id = response.data.id; const permission = response.data.permission localStorage.setItem("token", token); localStorage.setItem("id",id); localStorage.setItem("permission",permission) this.$message({ message: "Logged in successfully!", type: "success", }); this.$router.push({ name: "MinesApp" }); } catch (error) { console.log(error); this.$message({ message: "Invalid credentials!", type: "error", }); } } else { this.$message({ message: "Invalid credentials!", type: "error", }); return false; } }); }, }, };什么意思
这段代码是一个Vue.js组件,用于用户登录和注册功能。其中包含两个数据对象:loginForm和registerForm,用于保存用户输入的用户名和密码。同时,还有两个方法:submitForm和submitRegisterForm,分别用于提交登录和注册表单。这里使用了axios库来发送HTTP请求,并根据服务器返回结果来处理登录和注册的成功或失败情况。当用户注册成功后,会自动登录并跳转到应用主页面。
优化代码 methods: { onSubmit(values) { console.log("submit", values); if(values.username === this.$username && values.password === this.$password) { localStorage.setItem("Token", false) this.$router.push({path: '/'}) } } }
这段代码可以进行以下优化:
1. 将 `values` 对象中的属性解构出来,以便更方便地获取 `username` 和 `password` 属性的值。
2. 将对 `$username` 和 `$password` 的访问改为对组件实例中的 `username` 和 `password` 属性的访问,以避免使用 `$` 符号。
3. 将 `localStorage.setItem` 方法的值改为 `true`,以表示用户已经通过身份验证,并将该值的键名改为一个常量,以避免硬编码。
4. 在调用 `$router.push` 方法时,使用命名路由(name)而不是路径(path),以提高代码的可读性。
优化后的代码如下所示:
```
const TOKEN_KEY = "Token";
methods: {
onSubmit({ username, password }) {
console.log("submit", { username, password });
if (username === this.username && password === this.password) {
localStorage.setItem(TOKEN_KEY, true);
this.$router.push({ name: "home" });
}
}
}
```
其中,假设在路由配置中有一个名为 `home` 的路由项。
阅读全文
相关推荐
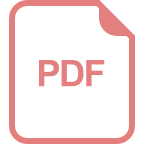



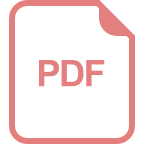
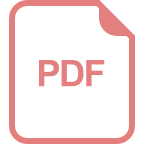
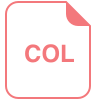
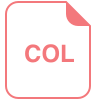
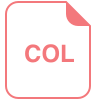






