yolo人脸检测python
时间: 2023-10-16 11:02:51 浏览: 64
YOLO(You Only Look Once)是一种先进的物体检测算法,可以应用于人脸检测。Python是一种流行的编程语言,可以用于实现YOLO人脸检测。
YOLO人脸检测的实现步骤如下:
1. 下载并安装YOLO的Python库以及相关依赖项,如OpenCV和Numpy。
2. 获取训练好的YOLO人脸检测模型,可以在互联网上找到已经训练好的模型,也可以自己训练一个模型。
3. 导入所需库和模型文件,在Python中加载YOLO模型。
4. 通过OpenCV库读取待检测的图像或视频,并进行预处理,如调整图像尺寸和像素值的归一化。
5. 将预处理后的图像传入YOLO模型进行人脸检测,模型将返回检测到的人脸的位置和概率。
6. 根据返回的人脸位置信息,在图像上绘制人脸的边界框和标签,并显示检测结果。
7. 可以选择保存检测结果或者将结果实时显示在屏幕上。
8. 结束检测后释放资源,关闭程序。
通过使用YOLO人脸检测算法,结合Python编程语言的强大功能,我们可以快速高效地进行人脸检测。这对于许多应用场景中的人脸识别、人脸表情分析、人脸替换等任务具有重要意义。
相关问题
yolo人脸检测代码
以下是使用YOLOv3算法进行人脸检测的Python代码:
```python
import cv2
import numpy as np
# 加载YOLOv3模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 获取输出层的名称
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 加载物体类别名称
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 定义颜色列表,用于将不同的框标记为不同的颜色
colors = np.random.uniform(0, 255, size=(len(classes), 3))
# 加载图像
img = cv2.imread("test.jpg")
height, width, channels = img.shape
# 对输入图像进行预处理
blob = cv2.dnn.blobFromImage(img, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
# 将预处理后的图像输入到神经网络中
net.setInput(blob)
outs = net.forward(output_layers)
# 初始化检测结果列表
class_ids = []
confidences = []
boxes = []
# 对输出层进行解析,获取检测结果
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5 and class_id == 0: # 只保留置信度大于0.5的人脸检测结果
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
# 计算框的左上角坐标
x = int(center_x - w / 2)
y = int(center_y - h / 2)
# 将检测结果保存到列表中
class_ids.append(class_id)
confidences.append(float(confidence))
boxes.append([x, y, w, h])
# 对检测结果进行非极大值抑制
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 在图像上标记检测结果
font = cv2.FONT_HERSHEY_PLAIN
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
color = colors[class_ids[i]]
cv2.rectangle(img, (x, y), (x + w, y + h), color, 2)
cv2.putText(img, label, (x, y - 5), font, 1, color, 2)
# 显示标记后的图像
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,YOLOv3算法需要预训练的权重文件和配置文件,可以在官网下载。此外,由于YOLOv3算法是基于Darknet框架实现的,因此需要使用OpenCV的dnn模块进行加载和调用。
YOLO人脸检测算法怎么用
YOLO(You Only Look Once)是一种实时目标检测算法,可以用于人脸检测。以下是使用YOLO人脸检测算法的步骤:
1. 输入图像:将要检测人脸的图像输入到YOLO算法中。
2. 网络预测:通过对输入图像的卷积和池化操作,YOLO会将图像分成多个网格,并对每个网格进行预测,预测出该网格内是否包含人脸以及人脸的位置。
3. 预测后处理:将所有网格的预测结果进行后处理,包括去除重复的预测结果、筛选出置信度高的预测结果等。
4. 输出结果:输出所有检测到的人脸的位置和大小。
以下是使用Python和OpenCV库实现YOLO人脸检测的代码示例:
```
import cv2
yolo = cv2.dnn.readNet("yolov3-face.cfg", "yolov3-wider_16000.weights")
conf_threshold = 0.5
nms_threshold = 0.4
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
height, width, _ = frame.shape
blob = cv2.dnn.blobFromImage(frame, 1/255, (416, 416), swapRB=True)
yolo.setInput(blob)
output_layers = yolo.getUnconnectedOutLayersNames()
outputs = yolo.forward(output_layers)
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > conf_threshold and class_id == 0:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w/2)
y = int(center_y - h/2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
indices = cv2.dnn.NMSBoxes(boxes, confidences, conf_threshold, nms_threshold)
for i in indices:
i = i[0]
x, y, w, h = boxes[i]
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.imshow("Face Detection", frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
在代码中,首先使用`cv2.dnn.readNet()`函数读取预训练的YOLO模型和权重文件,然后设置置信度和非最大值抑制的阈值。接着,使用`cv2.VideoCapture()`函数获取摄像头的视频流,并在每一帧图像中进行人脸检测。最后,使用`cv2.rectangle()`函数在检测到的人脸周围画一个矩形框,显示检测结果。
相关推荐
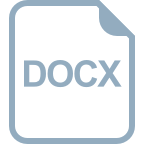
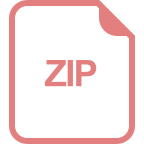
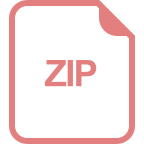












