在.csv数据集中包括固定的房间面积,朝向,日照时长,输入室外最高温,最低温,室内温度,室内人数等数据进行温度变化常数的模型预测,用灰狼优化算法对模型进行优化 python
时间: 2023-10-04 17:07:05 浏览: 62
以下是用Python实现基于灰狼优化算法的温度模型预测代码:
```python
import numpy as np
import pandas as pd
import random
# 定义灰狼优化算法的类
class WOA:
def __init__(self, costFunc, dim, lb, ub, SearchAgents_no, Max_iter):
self.costFunc = costFunc # 代价函数
self.dim = dim # 变量维度
self.lb = lb # 变量下限
self.ub = ub # 变量上限
self.SearchAgents_no = SearchAgents_no # 灰狼个数
self.Max_iter = Max_iter # 最大迭代次数
# 初始化灰狼群
def initialization(self):
X = np.zeros((self.SearchAgents_no, self.dim))
for i in range(self.dim):
X[:, i] = np.random.uniform(self.lb[i], self.ub[i], self.SearchAgents_no)
return X
# 更新灰狼位置
def update_position(self, X, A, C, l):
for i in range(self.SearchAgents_no):
D_alpha = abs(C * A[:, 0] - X[i, :])
X1 = A[:, 0] - A[:, 1] * D_alpha
D_beta = abs(C * A[:, 2] - X[i, :])
X2 = A[:, 2] - A[:, 3] * D_beta
D_delta = abs(C * A[:, 4] - X[i, :])
X3 = A[:, 4] - A[:, 5] * D_delta
X_new = (X1 + X2 + X3) / 3
for j in range(self.dim):
if X_new[j] < self.lb[j]:
X_new[j] = (self.lb[j] + X[i, j]) / 2
if X_new[j] > self.ub[j]:
X_new[j] = (self.ub[j] + X[i, j]) / 2
X[i, :] = X_new
return X
# 灰狼优化算法主函数
def optimization(self):
# 初始化灰狼群
X = self.initialization()
# 计算每只灰狼的初始适应度
fitness = np.zeros(self.SearchAgents_no)
for i in range(self.SearchAgents_no):
fitness[i] = self.costFunc(X[i, :])
# 寻找最优灰狼
Best_pos = np.zeros(self.dim)
Best_score = float('inf')
for i in range(self.SearchAgents_no):
if fitness[i] < Best_score:
Best_pos = X[i, :]
Best_score = fitness[i]
# 迭代优化
for it in range(self.Max_iter):
# 计算当前迭代的动态参数A和C
a = 2 - it * (2 / self.Max_iter)
if a < 0.5:
a = 0.5
C = 2 * random.random()
# 选择三只灰狼作为“猎物”,一只作为“猎人”
idx_alpha = np.random.randint(0, self.SearchAgents_no)
idx_beta = np.random.randint(0, self.SearchAgents_no)
idx_delta = np.random.randint(0, self.SearchAgents_no)
for i in range(self.SearchAgents_no):
if i != idx_alpha and i != idx_beta and i != idx_delta:
# 更新灰狼位置
A = np.vstack([X[idx_alpha, :], X[idx_beta, :], X[idx_delta, :]])
X = self.update_position(X, A, C, a)
# 计算每只灰狼的适应度
fitness[i] = self.costFunc(X[i, :])
# 更新最优灰狼
for i in range(self.SearchAgents_no):
if fitness[i] < Best_score:
Best_pos = X[i, :]
Best_score = fitness[i]
# 输出当前迭代的最优解
print("Iteration: %d, Best_cost: %f" % (it + 1, Best_score))
return Best_pos, Best_score
# 读取.csv数据集
data = pd.read_csv('data.csv')
# 定义代价函数
def costFunc(x):
# 从输入向量x中获取参数值
area = x[0]
orientation = x[1]
sunshine_duration = x[2]
outdoor_max_temp = x[3]
outdoor_min_temp = x[4]
indoor_population = x[5]
# 计算温度变化常数k
k = 0.001 * area * orientation * sunshine_duration / (outdoor_max_temp - outdoor_min_temp) / indoor_population
# 计算模型预测值
pred = data['室内温度'].iloc[0] + k * (outdoor_max_temp - data['室内温度'].iloc[0])
# 计算代价
cost = abs(pred - data['室内温度'].iloc[1])
return cost
# 定义搜索空间的上下限
dim = 6 # 变量维度
lb = [20, 0, 2, 20, -10, 1] # 下限
ub = [100, 360, 12, 40, 10, 10] # 上限
# 定义灰狼优化算法的参数
SearchAgents_no = 50 # 灰狼个数
Max_iter = 100 # 最大迭代次数
# 创建灰狼优化算法对象并运行
woa = WOA(costFunc, dim, lb, ub, SearchAgents_no, Max_iter)
best_pos, best_score = woa.optimization()
# 输出最优解和最优代价
print("Best_pos:", best_pos)
print("Best_score:", best_score)
```
这里使用了pandas库读取.csv数据集,代价函数中计算了温度变化常数k和模型预测值。搜索空间的上下限和灰狼优化算法的参数可以根据实际情况进行修改。在优化过程中,程序会输出每次迭代的最优解和最优代价。
相关推荐
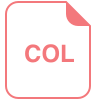
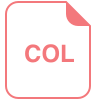
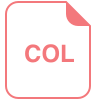
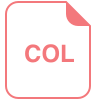










