有一个邻接表存储的图G,分别用C语言设计实现以下要求的算法:求出图中每个顶点的出度;计算图中出度为0的顶点数。
时间: 2024-04-30 07:17:16 浏览: 6
好的,您的第一个问题是关于邻接表存储的图的算法。针对这个问题,我可以提供以下代码实现:
```
// 定义邻接表存储结构
typedef struct Node {
int vertex; // 边的终止点
struct Node * next; // 指向下一条边的指针
} Node;
typedef struct Graph {
Node * adjList[MAX_VERTICES]; // 邻接表数组
int outDegree[MAX_VERTICES]; // 每个顶点的出度
int numVertices; // 图中顶点数
int numEdges; // 图中边数
} Graph;
// 计算每个顶点的出度
void calculateOutDegree(Graph * graph) {
// 初始化每个顶点的出度为0
for (int i = 0; i < graph->numVertices; i++) {
graph->outDegree[i] = 0;
}
// 遍历邻接表,统计每个顶点的出度
for (int i = 0; i < graph->numVertices; i++) {
Node * curr = graph->adjList[i];
while (curr != NULL) {
graph->outDegree[i]++;
curr = curr->next;
}
}
}
// 计算出度为0的顶点数
int countVerticesWithOutDegreeZero(Graph * graph) {
int count = 0;
for (int i = 0; i < graph->numVertices; i++) {
if (graph->outDegree[i] == 0) {
count++;
}
}
return count;
}
```
此处的算法包括两个函数:`calculateOutDegree`和`countVerticesWithOutDegreeZero`。
`calculateOutDegree`函数用于计算图中每个顶点的出度。该函数首先将每个顶点的出度初始化为0,然后遍历所有邻接表,统计每个顶点的出度。
`countVerticesWithOutDegreeZero`函数用于计算图中出度为0的顶点数。该函数遍历所有顶点的出度,统计出度为0的顶点数并返回。
通过调用这两个函数,您可以轻松地解决您的问题。
相关推荐
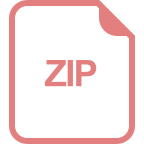
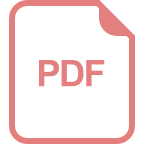














