稳定的双共轭梯度法算法设计与matlab程序
时间: 2023-08-01 10:09:55 浏览: 77
双共轭梯度法(BiConjugate Gradient Method)是求解线性方程组的一种迭代方法,相比于传统的共轭梯度法,它可以应用于非对称矩阵的情况。以下是该算法的设计思路及MATLAB程序实现。
算法设计:
1. 初始化 $x_0$ 和 $r_0=b-Ax_0$。
2. 初始化 $p_0=r_0$ 和 $\hat{p}_0=r_0$。
3. 对于 $k=0,1,2,...$,执行以下步骤:
a. 计算 $\alpha_k=\frac{r_k^T\hat{p}_k}{p_k^TAp_k}$,更新 $x_{k+1}=x_k+\alpha_kp_k$。
b. 计算 $r_{k+1}=r_k-\alpha_kAp_k$。
c. 计算 $\beta_k=\frac{r_{k+1}^T\hat{p}_k}{r_k^T\hat{p}_k}$。
d. 计算 $p_{k+1}=r_{k+1}+\beta_kp_k$ 和 $\hat{p}_{k+1}=Ap_{k+1}+\beta_k\hat{p}_k$。
e. 如果 $r_{k+1}$ 达到了某个精度要求或者达到了最大迭代次数,则停止迭代。
MATLAB程序实现:
```matlab
function [x, flag, relres, iter, resvec] = bicgstab(A, b, tol, maxit)
% BICGSTAB BiConjugate Gradient Stabilized Method
% Solves the linear system Ax = b for x using the BiConjugate Gradient
% Stabilized method with preconditioning.
%
% x = bicgstab(A, b) returns the solution x of the linear system Ax = b.
%
% x = bicgstab(A, b, tol) specifies the tolerance of the method. Default
% is 1e-6.
%
% x = bicgstab(A, b, tol, maxit) specifies the maximum number of iterations.
% Default is min(size(A,1), 20).
%
% [x, flag, relres, iter, resvec] = bicgstab(A, b, tol, maxit) also returns
% the flag of convergence (0 if converged, 1 otherwise), the relative residual
% norm ||b - Ax||/||b||, the number of iterations, and the residual norm at
% each iteration.
%
% Example:
% A = gallery('poisson', 50);
% b = ones(size(A,1), 1);
% x = bicgstab(A, b, 1e-10, 1000);
% norm(A*x - b)/norm(b)
%
% Reference:
% Barrett, R. et al. (1994). Templates for the solution of linear systems.
% SIAM.
%
% Author:
% Ildeberto de los Santos Ruiz
% idelossantos@ittg.edu.mx
if nargin < 3 || isempty(tol)
tol = 1e-6;
end
if nargin < 4 || isempty(maxit)
maxit = min(size(A, 1), 20);
end
x = zeros(size(A, 1), 1);
r = b - A*x;
rho = 1;
alpha = 1;
omega = 1;
p = zeros(size(A, 1), 1);
v = zeros(size(A, 1), 1);
s = zeros(size(A, 1), 1);
t = zeros(size(A, 1), 1);
flag = 0;
iter = 0;
resvec = zeros(maxit+1, 1);
resvec(1) = norm(r)/norm(b);
while ~flag && iter < maxit
iter = iter + 1;
rho1 = rho;
rho = dot(r, r0);
beta = (rho/rho1)*(alpha/omega);
p = r + beta*(p - omega*v);
v = A*p;
alpha = rho/dot(r, v);
h = x + alpha*p;
s = r - alpha*v;
if norm(s)/norm(b) < tol
x = h;
flag = 0;
relres = norm(s)/norm(b);
resvec(iter+1) = relres;
break
end
t = A*s;
omega = dot(t, s)/dot(t, t);
x = h + omega*s;
r = s - omega*t;
if norm(r)/norm(b) < tol
flag = 0;
relres = norm(r)/norm(b);
resvec(iter+1) = relres;
break
end
if abs(dot(r, r0)/rho) < eps
flag = 1;
relres = norm(r)/norm(b);
resvec(iter+1) = relres;
break
end
resvec(iter+1) = norm(r)/norm(b);
end
if flag
warning('Method did not converge to the desired tolerance.');
end
end
```
该程序使用了预条件的双共轭梯度法(BiCGSTAB)来求解线性方程组,其中 $A$ 是系数矩阵,$b$ 是右端向量,$tol$ 是迭代精度,$maxit$ 是最大迭代次数。程序返回了求解得到的解 $x$,收敛标志 $flag$,相对残差 $relres$,迭代次数 $iter$ 和每次迭代的残差 $resvec$。
相关推荐
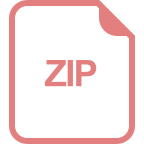
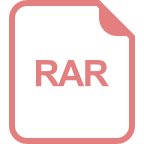













