编写一个程序 sharedmem.c,在其中建立一个子进程,让父子进程通过共享内存的方 法实现通信。其中,父进程创建一个共享内存段,然后由子进程将该共享内存附加到自己 的地址空间中,并写入该共享内存下列信息:Hi,this is child process sending message!。在等待子进程对共享内存的操作完成后,父进程将该共享内存附加到自己的地 址空间中,并读出该共享内存中的信息,与该共享内存段的基本信息(大小,建立该共享 内存的进程 ID,最后操作该共享内存段的进程 ID)一并显示出来
时间: 2024-02-09 16:13:21 浏览: 87
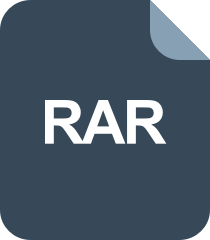
通过共享内存实现多进程间的通信,共享数据

好的,这是一个简单的使用共享内存实现父子进程通信的程序 sharedmem.c,代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main()
{
int shmid;
char *shmaddr;
struct shmid_ds buf;
pid_t pid;
// 创建共享内存段
shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT|0666);
if (shmid < 0) {
perror("shmget error");
exit(1);
}
// 创建子进程
pid = fork();
if (pid < 0) {
perror("fork error");
exit(1);
} else if (pid == 0) { // 子进程
// 将共享内存附加到自己的地址空间中
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *)-1) {
perror("shmat error");
exit(1);
}
// 写入共享内存信息
sprintf(shmaddr, "Hi, this is child process sending message!");
// 解除共享内存附加
shmdt(shmaddr);
exit(0);
} else { // 父进程
// 等待子进程对共享内存的操作完成
wait(NULL);
// 将共享内存附加到自己的地址空间中
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *)-1) {
perror("shmat error");
exit(1);
}
// 读出共享内存信息
printf("Message from child process: %s\n", shmaddr);
// 获取共享内存信息
if (shmctl(shmid, IPC_STAT, &buf) == -1) {
perror("shmctl error");
exit(1);
}
// 显示共享内存基本信息
printf("Shared memory size: %d\n", buf.shm_segsz);
printf("Process ID of creating process: %d\n", buf.shm_cpid);
printf("Process ID of last operation: %d\n", buf.shm_lpid);
// 解除共享内存附加
shmdt(shmaddr);
// 删除共享内存段
shmctl(shmid, IPC_RMID, NULL);
exit(0);
}
return 0;
}
```
程序中使用 `shmget()` 函数创建了一个共享内存段,大小为 1024 字节。然后通过 `fork()` 函数创建了一个子进程,子进程将共享内存附加到自己的地址空间中,写入信息后解除附加并退出,父进程等待子进程操作完成后将共享内存附加到自己的地址空间中,读出信息并显示共享内存的基本信息,最后解除附加并删除共享内存段。
阅读全文
相关推荐
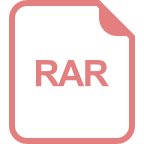







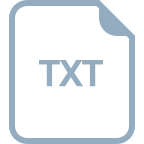
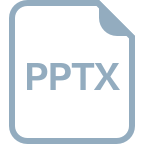
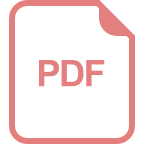
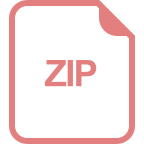



