使用c语言实现稀疏矩阵的存储表示(十字链表),并且实现矩阵的转置。
时间: 2024-11-24 16:42:47 浏览: 35
在C语言中,稀疏矩阵的十字链表存储通常采用三元组结构,即`struct`来表示非零元素。每个非零元素由行索引、列索引和对应的值组成。这里是一个简单的十字链表结构示例:
```c
typedef struct Node {
int row;
int col;
double value;
struct Node* next; // 指向下一个非零元素的指针
} SparseMatrixNode;
typedef struct SparseMatrix {
SparseMatrixNode* head; // 链表头部
int numRows;
int numCols;
} SparseMatrix;
```
矩阵转置的操作可以遍历原矩阵的每一个非零元素,并将其对应到新矩阵的相应位置上。以下是转置过程的一个简单函数实现:
```c
void transpose(SparseMatrix* src, SparseMatrix* dst) {
if (src->numRows != dst->numCols || src->head == NULL) return;
SparseMatrixNode* current = src->head;
while (current != NULL) {
SparseMatrixNode* new_node = malloc(sizeof(SparseMatrixNode));
new_node->row = current->col;
new_node->col = src->numCols - current->row - 1;
new_node->value = current->value;
new_node->next = dst->head;
dst->head = new_node;
current = current->next;
}
}
```
在这个函数中,我们假设原矩阵是方阵,并且已经按照行优先的顺序存储。
阅读全文
相关推荐


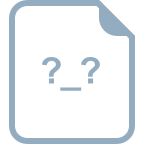












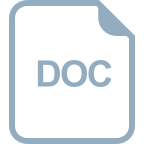

