创建Employee的结构体,包含成员变量name和department,func,s输入vector<Employee>参数,返回vector<std::string>,按照部门员工人数从高到低的顺序列出所有部门名称
时间: 2024-10-28 13:13:17 浏览: 30
在C++中,你可以创建一个名为`Employee`的结构体,并使用STL容器如`std::vector`来存储员工信息。首先,我们需要定义结构体`Employee`,包含姓名(name)、部门(department)和函数(func)。
```cpp
struct Employee {
std::string name;
std::string department;
// 如果有函数,可以是一个虚函数用于排序或处理
virtual void process() {}
};
```
接下来,你可以创建一个辅助函数`listDepartmentsByCount`,它接收一个`std::vector<Employee>`作为参数,计算每个部门的员工数量,并按数量降序排列部门名:
```cpp
std::vector<std::pair<std::string, int>> countEmployeesByDepartment(const std::vector<Employee>& employees) {
std::map<std::string, int> departmentCounts;
for (const auto& employee : employees) {
departmentCounts[employee.department]++;
}
// 使用std::vector<std::pair>存储部门名和计数,然后排序
std::vector<std::pair<std::string, int>> sortedCounts(departmentCounts.begin(), departmentCounts.end());
std::sort(sortedCounts.begin(), sortedCounts.end(), [](const auto &a, const auto &b) { return a.second > b.second; });
// 将排序后的部门名和计数转换为字符串列表并返回
std::vector<std::string> result;
for (const auto &count : sortedCounts) {
result.push_back(count.first + ": " + to_string(count.second));
}
return result;
}
// 调用该函数并返回结果
std::vector<std::string> listDepartmentsByDescendingCount(const std::vector<Employee>& employees) {
return listDepartmentsByCount(employees);
}
```
当你需要列出各部门员工人数时,只需传入`Employee`的实例向量即可:
```cpp
int main() {
std::vector<Employee> employees = {/* ... */}; // 初始化员工向量
std::vector<std::string> departments = listDepartmentsByDescendingCount(employees);
for (const auto &department : departments) {
std::cout << department << "\n";
}
return 0;
}
```
阅读全文
相关推荐
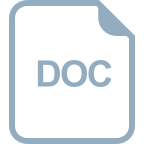
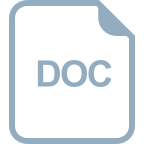
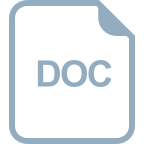















