撤销操作 python代码
时间: 2023-10-12 07:11:31 浏览: 32
Python中的撤销操作通常使用栈(Stack)来实现。栈是一种先进后出(Last In First Out,LIFO)的数据结构,可以用列表(List)来模拟。
下面是一个简单的示例代码,实现了栈的基本操作和撤销操作:
```python
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
def peek(self):
if not self.is_empty():
return self.items[-1]
def size(self):
return len(self.items)
class Editor:
def __init__(self):
self.text = ""
self.undo_stack = Stack()
self.redo_stack = Stack()
def add_text(self, text):
self.undo_stack.push(self.text)
self.text += text
def delete_text(self, n):
self.undo_stack.push(self.text)
self.text = self.text[:-n]
def undo(self):
if not self.undo_stack.is_empty():
self.redo_stack.push(self.text)
self.text = self.undo_stack.pop()
def redo(self):
if not self.redo_stack.is_empty():
self.undo_stack.push(self.text)
self.text = self.redo_stack.pop()
```
在这个示例代码中,我们定义了一个名为`Editor`的类,它包含了一个`text`字符串和两个栈:`undo_stack`和`redo_stack`,分别用于保存撤销和重做操作的历史记录。在`add_text`和`delete_text`方法中,我们将当前的`text`添加到`undo_stack`中,以便在撤销操作时能够恢复到之前的状态。在`undo`和`redo`方法中,我们分别从`undo_stack`和`redo_stack`中取出最新的历史记录,并更新`text`字符串。
我们可以通过以下代码来测试这个示例:
```python
editor = Editor()
editor.add_text("Hello")
editor.add_text(" world")
print(editor.text) # Hello world
editor.undo()
print(editor.text) # Hello
editor.undo()
print(editor.text) #
editor.redo()
print(editor.text) # Hello
editor.add_text(", how are you?")
print(editor.text) # Hello, how are you?
editor.redo()
print(editor.text) # Hello, how are you?
```
在上面的测试代码中,我们首先添加了两个字符串,然后进行了两次撤销,再进行一次重做,最后添加了另一个字符串并进行一次重做。如果一切正常,输出应该为:
```
Hello world
Hello
Hello world
Hello, how are you?
```
相关推荐
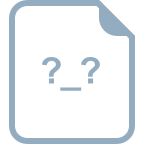
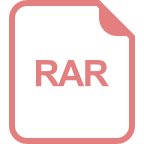
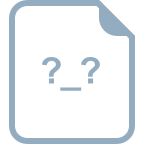














