在不同主机下,设置3台usrp b210用pps设置同步采集,用C++代码实现
时间: 2024-05-08 15:16:43 浏览: 176
为了实现3台usrp b210的pps同步采集,需要进行以下步骤:
1. 确定主机之间的时钟同步:在不同的主机之间,需要确保时钟同步。可以使用ntp或其他时钟同步协议来实现。
2. 设置usrp b210的pps输出:在每台usrp b210上,需要将pps输出连接到一个共同的信号源,如GPS或外部时钟源。可以使用UHD API中的函数uhd::usrp::multi_usrp::set_time_source()来设置pps输出。
3. 设置usrp b210的pps输入:在每台usrp b210上,需要将pps输入连接到共同的信号源。可以使用UHD API中的函数uhd::usrp::multi_usrp::set_time_source()来设置pps输入。
4. 编写C代码实现采集:使用UHD API中的函数uhd::usrp::multi_usrp::recv()来采集数据。在调用recv()函数之前,需要先使用uhd::usrp::multi_usrp::set_time_now()函数将所有设备的时间设置为相同的时间戳(以微秒为单位)。
下面是一个简单的C程序示例,演示如何使用UHD API在3台usrp b210之间同步采集数据:
```c
#include <uhd/utils/thread.hpp>
#include <uhd/usrp/multi_usrp.hpp>
#include <iostream>
#include <vector>
int main()
{
// Define the IP addresses of the 3 USRP B210 devices
std::vector<std::string> device_addrs = {"addr1", "addr2", "addr3"};
// Create a vector of USRP devices
std::vector<uhd::usrp::multi_usrp::sptr> usrp_devices;
// Create a USRP device object for each IP address and add it to the vector
for(const auto& addr : device_addrs)
{
uhd::usrp::multi_usrp::sptr usrp = uhd::usrp::multi_usrp::make(addr);
usrp_devices.push_back(usrp);
}
// Set the PPS source to external on all devices
for(const auto& usrp : usrp_devices)
{
usrp->set_time_source("external");
}
// Set the time on all devices to the same timestamp (in microseconds)
uhd::time_spec_t start_time = uhd::time_spec_t(0.0);
for(const auto& usrp : usrp_devices)
{
usrp->set_time_now(start_time);
}
// Configure the receive stream parameters
uhd::stream_args_t stream_args("fc32");
stream_args.channels = {0};
stream_args.cpu_format = "sc16";
stream_args.otw_format = "sc16";
stream_args.args = "";
// Create a receive stream object for each device
std::vector<uhd::rx_streamer::sptr> rx_streams;
for(const auto& usrp : usrp_devices)
{
rx_streams.push_back(usrp->get_rx_stream(stream_args));
}
// Start the receive streams on all devices
for(const auto& rx_stream : rx_streams)
{
rx_stream->start();
}
// Start the receive threads on all devices
std::vector<uhd::thread::sptr> rx_threads;
for(const auto& rx_stream : rx_streams)
{
uhd::thread::sptr thread = uhd::rx_streamer::thread_func(rx_stream, uhd::cpu_affinity_t());
rx_threads.push_back(thread);
}
// Wait for the receive threads to finish
for(const auto& thread : rx_threads)
{
thread->join();
}
// Stop the receive streams on all devices
for(const auto& rx_stream : rx_streams)
{
rx_stream->stop();
}
return 0;
}
```
注意:以上示例只是一个简单的示例,只涉及到一些基本的UHD函数和参数。实际使用时,需要根据实际情况进行更详细和复杂的配置和设置。
阅读全文
相关推荐
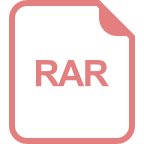
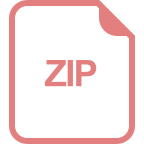














