使用C语言写一个普通算术代表式,其中操作数为整数,运算符不少8个(含一对括号),四则运算至少各一个,改成后缀表达式,输入后缀表达式,实现后缀表达式的计算。
时间: 2024-10-12 22:07:25 浏览: 21
在C语言中,我们可以编写一个程序来实现将前缀(也称为逆波兰表示法,即运算符位于操作数之后)到后缀(常规的数学表达式形式)的转换,并计算后缀表达式的值。这是一个简单的步骤过程:
首先,我们需要创建一个栈来存储运算符,然后遍历给定的前缀表达式。遇到数字就把它压入结果数组;遇到左括号就压入栈;遇到右括号时,就从栈顶弹出运算符直到遇到左括号,把它们依次添加到结果数组,然后继续处理剩余的括号。
当遍历完整个表达式后,如果栈非空,说明还有未处理的运算符,需要计算这些运算符并替换结果数组相应的位置。最后,我们只需要按照数组顺序读取和计算,就可以得到后缀表达式的值。
下面是C语言的一个简单示例,这里没有包含完整的错误处理和用户输入部分,只展示了核心算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义栈结构和相关的函数
typedef struct {
char *top;
int capacity;
} Stack;
Stack* createStack(int cap) {
Stack *stack = (Stack*)malloc(sizeof(Stack));
stack->top = NULL;
stack->capacity = cap;
return stack;
}
void push(Stack *stack, char op) {
if(stack->top == stack->capacity) {
// 栈满,需要扩展
stack->capacity *= 2;
stack->top = realloc(stack->top, stack->capacity);
}
stack->top++;
*stack->top = op;
}
char pop(Stack *stack) {
if(stack->top == NULL)
return '\0'; // 表示栈空
char op = stack->top--;
return op;
}
int evaluatePostfix(char *postfixExp) {
Stack *stack = createStack(10);
char currentChar;
for(int i = 0; postfixExp[i] != '\0'; ++i) {
currentChar = postfixExp[i];
if(isdigit(currentChar)) { // 遇到数字直接压入栈
printf("%d", currentChar - '0'); // 如果你需要,可以在这里做乘除运算
continue;
}
while(!isspace(stack->top) && precedence(stack->top) >= precedence(currentChar)) { // 考虑当前运算符的优先级
currentChar = pop(stack);
printf("%d", currentChar - '0');
}
push(stack, currentChar); // 将当前运算符压入栈
}
while(stack->top) {
currentChar = pop(stack);
printf("%d", currentChar - '0');
}
free(stack->top);
stack->top = NULL;
free(stack);
return 0; // 或者你可以返回最终结果,比如加总所有数字
}
// 假设 precedence 函数给出了运算符的优先级
int precedence(char op) {
// 根据实际运算符的优先级定义此函数
// 如:op('+') > op('*')
}
int main() {
char postfixExp[] = "2 3 + 4 *";
evaluatePostfix(postfixExp);
return 0;
}
```
在这个例子中,`evaluatePostfix`函数接收一个后缀表达式作为字符串参数,然后计算其值。你可以根据需要调整`precedence`函数来确定各个运算符的优先级。
阅读全文
相关推荐
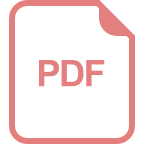
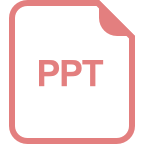
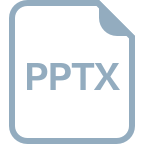
















