用c语言写算术表达式求值程序。演示程序和源程序已给出。用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/()。 编写源程序中的 double deal_exp (char *exp) 函数,对这个算术表达式求值,返回结果。不限制方法,你可以用“原始”的多遍扫描字符串,也可以用先进一点的栈
时间: 2023-12-17 09:04:30 浏览: 47
以下是用栈实现算术表达式求值的 C 语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define STACK_SIZE 100
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
void init_stack(Stack *s) {
s->top = -1;
}
void push(Stack *s, int item) {
if (s->top == STACK_SIZE - 1) {
printf("Stack overflow\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = item;
}
int pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
int peek(Stack *s) {
if (s->top == -1) {
printf("Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
int is_empty(Stack *s) {
return (s->top == -1);
}
int precedence(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
double evaluate_expression(char *exp) {
Stack operand_stack;
Stack operator_stack;
char *endptr;
char *p = exp;
init_stack(&operand_stack);
init_stack(&operator_stack);
while (*p != '\0') {
if (isspace(*p)) {
p++;
} else if (isdigit(*p)) {
int operand = strtol(p, &endptr, 10);
push(&operand_stack, operand);
p = endptr;
} else if (*p == '(') {
push(&operator_stack, '(');
p++;
} else if (*p == ')') {
while (peek(&operator_stack) != '(') {
char op = pop(&operator_stack);
int operand2 = pop(&operand_stack);
int operand1 = pop(&operand_stack);
switch (op) {
case '+':
push(&operand_stack, operand1 + operand2);
break;
case '-':
push(&operand_stack, operand1 - operand2);
break;
case '*':
push(&operand_stack, operand1 * operand2);
break;
case '/':
push(&operand_stack, operand1 / operand2);
break;
}
}
pop(&operator_stack);
p++;
} else {
while (!is_empty(&operator_stack) && peek(&operator_stack) != '(' && precedence(*p) <= precedence(peek(&operator_stack))) {
char op = pop(&operator_stack);
int operand2 = pop(&operand_stack);
int operand1 = pop(&operand_stack);
switch (op) {
case '+':
push(&operand_stack, operand1 + operand2);
break;
case '-':
push(&operand_stack, operand1 - operand2);
break;
case '*':
push(&operand_stack, operand1 * operand2);
break;
case '/':
push(&operand_stack, operand1 / operand2);
break;
}
}
push(&operator_stack, *p);
p++;
}
}
while (!is_empty(&operator_stack)) {
char op = pop(&operator_stack);
int operand2 = pop(&operand_stack);
int operand1 = pop(&operand_stack);
switch (op) {
case '+':
push(&operand_stack, operand1 + operand2);
break;
case '-':
push(&operand_stack, operand1 - operand2);
break;
case '*':
push(&operand_stack, operand1 * operand2);
break;
case '/':
push(&operand_stack, operand1 / operand2);
break;
}
}
return (double) peek(&operand_stack);
}
int main() {
char exp[100];
printf("Enter an arithmetic expression: ");
fgets(exp, sizeof(exp), stdin);
double result = evaluate_expression(exp);
printf("Result: %g\n", result);
return 0;
}
```
其中,`deal_exp` 函数被重命名为 `evaluate_expression`,并返回了 `double` 类型的结果。该函数用两个栈来实现算术表达式求值。一个栈(称为“操作数栈”)用于存储操作数,另一个栈(称为“运算符栈”)用于存储运算符。算法的基本思路是,从左到右扫描表达式的每个字符,如果是空格则跳过,如果是数字则将其转换成整数并压入操作数栈,如果是左括号则将其压入运算符栈,如果是右括号则弹出运算符栈中的运算符和操作数栈中的两个操作数,进行计算,并将计算结果压入操作数栈。如果是其他运算符,则不断弹出运算符栈中优先级大于等于该运算符的运算符,并从操作数栈中弹出两个操作数进行计算,然后将计算结果压入操作数栈。最后,当扫描完整个表达式后,将运算符栈中剩余的运算符和操作数栈中的操作数依次弹出进行计算即可。
相关推荐
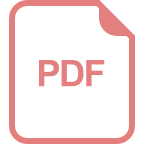













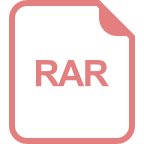