canvas.translate
时间: 2023-11-17 21:00:56 浏览: 34
canvas.translate()是HTML5中Canvas API提供的一个方法,用于对画布进行平移变换。它可以将画布在水平和垂直方向上移动指定的距离,从而改变画布中绘制图形的位置。在使用translate()方法时,需要注意它是针对画布进行平移变换的,因此在使用clearRect()等方法时也会受到平移的影响。为了清除画布上的内容,需要计算清楚清除矩形的起始坐标。可以参考上面提供的两个例子进行学习和理解。
相关问题
canvas.rotate
您好! 您可以使用Canvas的`rotate()`方法来旋转画布。这个方法接受一个参数,表示要旋转的角度(以弧度为单位)。例如,如果您想将画布顺时针旋转45度,可以使用以下代码:
```javascript
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
ctx.rotate(Math.PI / 4); // 45度角的弧度是π/4
// 在这之后进行绘制操作,绘制的内容将受到旋转的影响
```
请注意,旋转变换是相对于画布原点进行的,默认情况下原点位于左上角。如果您想要改变旋转的中心点,可以使用`translate()`方法将原点移到所需位置。
希望能对您有所帮助! 如有更多问题,请随时提问。
ctx.translate
ctx.translate是一个Canvas API中的方法,用于平移画布的原点到指定的位置。在上述代码中,ctx.translate(centerPosX, centerPosY)将画布的原点平移到画布的正中间位置。这样做的目的是为了让图片的旋转中心点位于画布的中心位置,从而实现图片的旋转效果。
#### 引用[.reference_title]
- *1* *2* *3* [Canvas 中translate与rotate详解](https://blog.csdn.net/zhehan54/article/details/88563867)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
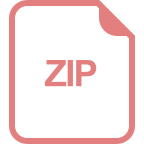
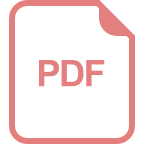












