public List<List<string>> GetDataCSV(string path) { string pattern = @"\d+"; List<List<string>> data = new List<List<string>>(); using(StreamReader sr = new StreamReader(@path, Encoding.GetEncoding("GB2312"))) { string nextline; MatchCollection myMatches; while((nextline = sr.ReadLine()) != null ) { myMatches = Regex.Matches(nextline, pattern); List<string> rowdata = new List<string>(); ; foreach(Match nextmatch in myMatches) { rowdata.Add(nextmatch.Value); } data.Add(rowdata); } //消除最后的空行 for(int i=data.Count-1;i>=0;i--) { if(data[i].Count ==0) { data.Remove(data[i]); } else { break; } } } return data; }解释每局什么意思
时间: 2024-01-29 12:03:22 浏览: 37
这段代码是一个 C# 函数,用于读取指定路径下的 CSV 文件,并将其中的数据以 List<List<string>> 的形式返回。下面是对这个函数的逐行解释:
```csharp
public List<List<string>> GetDataCSV(string path)
{
string pattern = @"\d+"; // 正则表达式,用于匹配数字
List<List<string>> data = new List<List<string>>(); // 创建二维 List,用于保存 CSV 中的数据
// 使用 StreamReader 打开指定路径下的文件
using (StreamReader sr = new StreamReader(@path, Encoding.GetEncoding("GB2312")))
{
string nextline; // 用于存储下一行的文本内容
MatchCollection myMatches; // 用于存储正则表达式匹配结果的集合
// 循环读取文件中的每一行
while ((nextline = sr.ReadLine()) != null)
{
// 使用正则表达式匹配当前行中的所有数字,并将它们添加到一个 List<string> 中
myMatches = Regex.Matches(nextline, pattern);
List<string> rowdata = new List<string>(); ;
foreach (Match nextmatch in myMatches)
{
rowdata.Add(nextmatch.Value);
}
// 将当前行的数据添加到二维 List 中
data.Add(rowdata);
}
// 消除最后的空行
for (int i = data.Count - 1; i >= 0; i--)
{
if (data[i].Count == 0)
{
data.Remove(data[i]);
}
else
{
break;
}
}
}
// 返回二维 List,其中每个元素都是一个包含数字的字符串 List
return data;
}
```
总体来说,这个函数的作用是读取 CSV 文件并将其中的数字数据保存到一个二维 List 中。具体实现过程如下:
1. 首先,定义一个正则表达式 pattern,用于匹配 CSV 文件中的数字。
2. 创建一个二维 List data,用于保存 CSV 文件中的数据。
3. 使用 StreamReader 打开指定路径下的 CSV 文件。
4. 循环读取文件中的每一行,使用正则表达式匹配当前行中的数字,并将它们添加到一个 List<string> rowdata 中。
5. 将 rowdata 添加到二维 List data 中。
6. 循环结束后,消除最后的空行。
7. 返回二维 List data,其中每个元素都是一个包含数字的字符串 List。
相关推荐
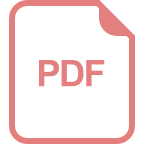
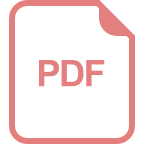
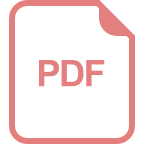










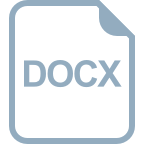
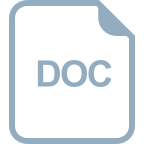
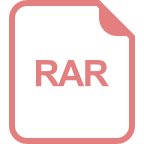
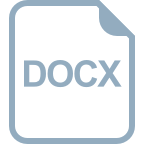
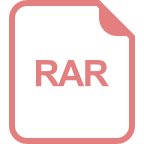
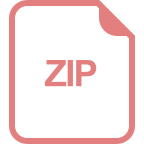