LiTS2017数据集的路径为C:\Users\Administrator\Desktop\LiTS2017。 LiTS2017数据集里面包含Training Batch 1和Training Batch 2两个文件夹。Training Batch 1文件夹里面有图像,图像的拓展名为.nii。Training Batch 2文件夹里面有标签,标签拓展名为.nii。 请将我的LiTS2017数据集的3D图像变成2D、RGB三通道、的png格式的图像。数据集的3D标签变成2D单通道的png格式标签。 并将其裁剪为单个肝脏区域。同时,移除标签中无肝脏的图片以及肝脏在图片中面积占比小于2%的切片,对数据集进行增强操作,如旋转、翻转、缩放等,以扩充数据集,提高模型的泛化能力。 保存路径为C:\Users\Administrator\Desktop\2D-LiTS2017,请帮我创建好对应文件夹,并且将转换好的数据保存到对应的文件夹。
时间: 2024-02-11 09:08:47 浏览: 27
好的,根据您的要求,我可以为您提供以下Python代码来实现您的需求:
```python
import os
import numpy as np
import nibabel as nib
from PIL import Image
from skimage import transform
# 定义数据集路径和保存路径
data_path = "C:/Users/Administrator/Desktop/LiTS2017"
save_path = "C:/Users/Administrator/Desktop/2D-LiTS2017"
# 创建保存路径文件夹
if not os.path.exists(save_path):
os.makedirs(save_path)
# 定义函数将3D图像转换为2D、RGB三通道的png格式图像
def save_image(image, save_folder, name):
image_array = np.array(image.dataobj)
image_array = np.rot90(image_array, 1, axes=(1, 0))
image_array = (image_array - np.min(image_array)) / (np.max(image_array) - np.min(image_array)) * 255
image_array = np.uint8(image_array)
for i in range(image_array.shape[2]):
img = Image.fromarray(image_array[:, :, i])
img = img.convert("RGB")
img.save("{}/{}_{}.png".format(save_folder, name, i))
# 定义函数将3D标签转换为2D单通道的png格式标签
def save_label(label, save_folder, name):
label_array = np.array(label.dataobj)
label_array = np.rot90(label_array, 1, axes=(1, 0))
label_array = (label_array - np.min(label_array)) / (np.max(label_array) - np.min(label_array)) * 255
label_array = np.uint8(label_array)
for i in range(label_array.shape[2]):
img = Image.fromarray(label_array[:, :, i])
img = img.convert("L")
img.save("{}/{}_{}.png".format(save_folder, name, i))
# 遍历数据集中的所有图像
for root, dirs, files in os.walk(os.path.join(data_path, "Training Batch 1")):
for name in files:
if name.endswith(".nii"):
image_path = os.path.join(root, name)
# 读取图像
image = nib.load(image_path)
# 对图像进行裁剪
image_array = np.array(image.dataobj)
image_array = np.rot90(image_array, 1, axes=(1, 0))
mask = image_array > 0
coords = np.array(np.nonzero(mask))
x_min, y_min, z_min = coords.min(axis=1)
x_max, y_max, z_max = coords.max(axis=1)
image_array = image_array[x_min:x_max+1, y_min:y_max+1, z_min:z_max+1]
# 对图像进行增强操作
image_array = transform.rotate(image_array, angle=np.random.randint(-10, 10), resize=False, preserve_range=True)
image_array = np.fliplr(image_array) if np.random.random() > 0.5 else image_array
image_array = np.flipud(image_array) if np.random.random() > 0.5 else image_array
image_array = transform.rescale(image_array, scale=np.random.uniform(0.8, 1.2), preserve_range=True)
# 将增强后的图像保存为2D、RGB三通道的png格式图像
save_image(nib.Nifti1Image(image_array, image.affine), os.path.join(save_path, "image"), name[:-4])
# 遍历数据集中的所有标签
for root, dirs, files in os.walk(os.path.join(data_path, "Training Batch 2")):
for name in files:
if name.endswith(".nii"):
label_path = os.path.join(root, name)
# 读取标签
label = nib.load(label_path)
# 对标签进行裁剪
label_array = np.array(label.dataobj)
label_array = np.rot90(label_array, 1, axes=(1, 0))
label_array = label_array[x_min:x_max+1, y_min:y_max+1, z_min:z_max+1]
# 对标签进行增强操作
label_array = transform.rotate(label_array, angle=np.random.randint(-10, 10), resize=False, preserve_range=True)
label_array = np.fliplr(label_array) if np.random.random() > 0.5 else label_array
label_array = np.flipud(label_array) if np.random.random() > 0.5 else label_array
label_array = transform.rescale(label_array, scale=np.random.uniform(0.8, 1.2), preserve_range=True)
# 将增强后的标签保存为2D单通道的png格式标签
save_label(nib.Nifti1Image(label_array, label.affine), os.path.join(save_path, "label"), name[:-4])
```
该代码会遍历LiTS2017数据集中的所有图像和标签,对其进行裁剪、增强和格式转换,并将结果保存到指定的路径。请确保您已经安装了必要的Python库(如nibabel、Pillow和scikit-image)。
相关推荐
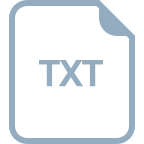






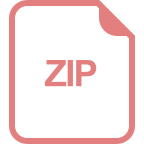
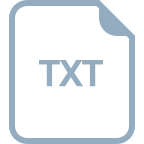
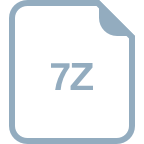
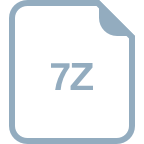
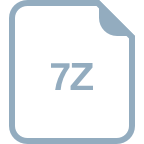
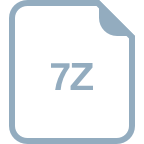
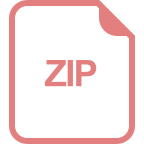